Quick example on how you can use jQuery’s $.map() function to grab a value from an array of JS objects. In the example I have an array of JS objects that contain days and prices, we want to extract a price for a specific day.
var dayArr = Array( { "day" : "day01", "price" : "$210" }, { "day" : "day02", "price" : "$220" }, { "day" : "day03", "price" : "$230" } );
var findDay = 'day02'; //find price for day 1
var price = $.map(dayArr, function(value, key) {
if (value.day == findDay)
{
return value.price;
}
});
console.log(price);
//output: $220
Frequently Asked Questions about jQuery Array Search
How does jQuery.inArray() function work?
The jQuery.inArray() function is a built-in method in jQuery that is used to search for a specified value within an array. If the value is found, it returns the index position of the value in the array. If the value is not found, it returns -1. The function takes two parameters: the value to search for, and the array in which to search. It’s important to note that the jQuery.inArray() function uses strict comparison (===), meaning both the type and the value we are comparing have to be the same for it to return true.
What is the difference between jQuery.inArray() and JavaScript’s indexOf()?
Both jQuery.inArray() and JavaScript’s indexOf() methods are used to find the position of a specific value in an array. However, there are some differences. jQuery.inArray() can work with both arrays and array-like objects, while indexOf() only works with arrays. Also, jQuery.inArray() can take an optional second argument that specifies the index at which to start the search, while indexOf() does not have this feature.
How can I use jQuery.inArray() to check if a value exists in an array?
To check if a value exists in an array using jQuery.inArray(), you can use the following code:var array = [1, 2, 3, 4, 5];
var value = 3;
if ($.inArray(value, array) != -1) {
alert(value + " exists in the array");
} else {
alert(value + " does not exist in the array");
}
In this code, if the value is found in the array, the function will return its index, which will always be a non-negative number. If the value is not found, the function will return -1.
Can jQuery.inArray() be used with multidimensional arrays?
No, jQuery.inArray() cannot be used directly with multidimensional arrays. It only works with one-dimensional arrays. If you need to search in a multidimensional array, you would need to loop through each sub-array and use jQuery.inArray() on each one.
How can I use jQuery.inArray() to find the index of a value in an array?
To find the index of a value in an array using jQuery.inArray(), you can use the following code:var array = [1, 2, 3, 4, 5];
var value = 3;
var index = $.inArray(value, array);
if (index != -1) {
alert("The index of " + value + " is " + index);
} else {
alert(value + " is not in the array");
}
In this code, if the value is found in the array, the function will return its index. If the value is not found, the function will return -1.
Can jQuery.inArray() be used with objects?
No, jQuery.inArray() cannot be used with objects. It only works with arrays and array-like objects. If you need to search in an object, you would need to convert the object to an array first, or use a different method.
How can I use jQuery.inArray() to remove a value from an array?
To remove a value from an array using jQuery.inArray(), you can use the following code:var array = [1, 2, 3, 4, 5];
var value = 3;
var index = $.inArray(value, array);
if (index != -1) {
array.splice(index, 1);
}
In this code, if the value is found in the array, the function will return its index, and then the splice() method is used to remove the value from the array.
Can jQuery.inArray() be used with strings?
Yes, jQuery.inArray() can be used with strings. It works the same way as with numbers. If the string is found in the array, the function will return its index. If the string is not found, the function will return -1.
How can I use jQuery.inArray() to find all occurrences of a value in an array?
jQuery.inArray() only returns the index of the first occurrence of a value in an array. If you need to find all occurrences, you would need to loop through the array and use jQuery.inArray() on each element.
Can jQuery.inArray() be used with arrays of objects?
No, jQuery.inArray() cannot be used directly with arrays of objects. It only works with arrays of primitive types (numbers, strings, etc.). If you need to search in an array of objects, you would need to loop through the array and compare the properties of each object.
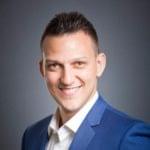
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.