//D = document
//W = window
//$ = jQuery
var contentArea = $(this),
wintop = contentArea.scrollTop(),
docheight = $(D).height(),
winheight = $(W).height(),
divheight = $('#content').height(),
scrollheight = $('#content')[0].scrollHeight,
scrolltrigger = 0.9;
console.log('wintop ' + wintop);
console.log('docheight ' + docheight);
console.log('winheight ' + winheight);
console.log('divheight ' + divheight);
console.log('scrollheight ' + scrollheight);
console.log((wintop + divheight)/scrollheight);
if (((wintop + divheight)/scrollheight) > scrolltrigger) {
// display scroll bar
}
Further reading:
http://manos.malihu.gr/jquery-custom-content-scroller
http://jscrollpane.kelvinluck.com/arrows.html
Frequently Asked Questions (FAQs) about jQuery Scrollbar
How can I add a scrollbar to a div using jQuery?
To add a scrollbar to a div using jQuery, you need to use the CSS property ‘overflow’. You can set this property to ‘auto’ or ‘scroll’. ‘Auto’ will add a scrollbar only when the content is larger than the div, while ‘scroll’ will always show a scrollbar. Here is a simple example:$("#divID").css("overflow", "auto");
In this example, replace “divID” with the ID of your div. This will add a scrollbar to the div when the content is larger than the div.
How can I scroll to a specific div using jQuery?
To scroll to a specific div using jQuery, you can use the ‘animate’ function along with the ‘scrollTop’ property. Here is an example:$('html, body').animate({
scrollTop: $("#divID").offset().top
}, 2000);
In this example, replace “divID” with the ID of your div. This will smoothly scroll to the div over a period of 2 seconds.
How can I add a scrollbar dynamically to a div using jQuery?
To add a scrollbar dynamically to a div using jQuery, you can use the ‘css’ function to change the ‘overflow’ property. Here is an example:$("#divID").css("overflow", "auto");
In this example, replace “divID” with the ID of your div. This will add a scrollbar to the div when the content is larger than the div.
How can I use jQuery to scroll to a div with a smooth animation?
To scroll to a div with a smooth animation using jQuery, you can use the ‘animate’ function along with the ‘scrollTop’ property. Here is an example:$('html, body').animate({
scrollTop: $("#divID").offset().top
}, 2000);
In this example, replace “divID” with the ID of your div. This will smoothly scroll to the div over a period of 2 seconds.
How can I add a horizontal scrollbar to a div using jQuery?
To add a horizontal scrollbar to a div using jQuery, you can use the ‘css’ function to change the ‘overflow-x’ property to ‘scroll’. Here is an example:$("#divID").css("overflow-x", "scroll");
In this example, replace “divID” with the ID of your div. This will add a horizontal scrollbar to the div.
How can I hide the scrollbar of a div using jQuery?
To hide the scrollbar of a div using jQuery, you can use the ‘css’ function to change the ‘overflow’ property to ‘hidden’. Here is an example:$("#divID").css("overflow", "hidden");
In this example, replace “divID” with the ID of your div. This will hide the scrollbar of the div.
How can I check if a div has a scrollbar using jQuery?
To check if a div has a scrollbar using jQuery, you can compare the div’s ‘scrollHeight’ or ‘scrollWidth’ with its ‘clientHeight’ or ‘clientWidth’. If ‘scrollHeight’ or ‘scrollWidth’ is greater, the div has a scrollbar. Here is an example:if ($("#divID")[0].scrollHeight > $("#divID").height()) {
// The div has a vertical scrollbar
}
In this example, replace “divID” with the ID of your div. This will check if the div has a vertical scrollbar.
How can I make the scrollbar of a div always visible using jQuery?
To make the scrollbar of a div always visible using jQuery, you can use the ‘css’ function to change the ‘overflow’ property to ‘scroll’. Here is an example:$("#divID").css("overflow", "scroll");
In this example, replace “divID” with the ID of your div. This will make the scrollbar of the div always visible.
How can I change the color of the scrollbar of a div using jQuery?
Changing the color of the scrollbar is not directly possible with jQuery, as it is a browser and operating system level element. However, you can use CSS to achieve this in some browsers like Chrome, Edge, or Safari. Here is an example:#divID::-webkit-scrollbar {
width: 12px;
}
#divID::-webkit-scrollbar-track {
background: #f1f1f1;
}
#divID::-webkit-scrollbar-thumb {
background: #888;
}
#divID::-webkit-scrollbar-thumb:hover {
background: #555;
}
In this example, replace “divID” with the ID of your div. This will change the color of the scrollbar of the div in Chrome, Edge, and Safari.
How can I scroll to the bottom of a div using jQuery?
To scroll to the bottom of a div using jQuery, you can use the ‘scrollTop’ function along with the ‘scrollHeight’ property. Here is an example:$("#divID").scrollTop($("#divID")[0].scrollHeight);
In this example, replace “divID” with the ID of your div. This will scroll to the bottom of the div.
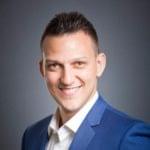
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.