Today, I wanted to use the Public Twitter Search API and grab the latest 5 tweets tagged “jquery4u”. Twitter provide a bunch of useful REST API Resources which you can make use of without a Twitter account or any authentication (oAuth or such) to grab data from Twitter. The Twitter Search API can be used to grab tweets using queries such as ones that have specific keywords, hashtags, users, mentions, phrases etc… Here is how you can do it.
The JavaScript/jQuery
Here we can access the public feed from the Twitter search API and specify a JSON callback for the data. Parameters: q = the search string (make sure it’s uri encoded) rpp = the number of tweets we want to get//get the JSON data from the Twitter search API
$.getJSON("http://search.twitter.com/search.json?q=jquery4u&rpp=5&callback=?", function(data)
{
//loop the tweets
$(data.results).each(function(i,v)
{
//...see full code below
}
}
The HTML
<div id="twitter-widget"><!-- tweets go here... --></div>
The CSS
.twitStream{
font-family: verdana;
font-size: 11px;
}
.twitStream a{
font-family: verdana;
font-size: 11px;
}
.tweet{
display: block;
padding: .4em;
margin: .4em 0;
}
.tweet-left{
float: left;
margin-right: 1em;
}
.tweet-left img{
border: 2px solid #000000;
}
.tweet p.text{
margin: 0;
padding: 0;
}
Full Code Listing
Here is the full JavaScript Object for the Twitter Widget./**
* JQUERY4U
*
* Displays the latest tweets.
*
* @author Sam Deering
* @copyright Copyright (c) 2012 JQUERY4U Pty Ltd
* @license http://jquery4u.com/license/
* @since Version 1.0
* @filesource js/jquery4u.twitter.js
*
*/
(function($,W,D)
{
W.JQUERY4U = W.JQUERY4U || {};
W.JQUERY4U.TWITTER = {
name: "JQUERY4U TWITTER",
init: function(wid)
{
//helper functions
String.prototype.linkify=function(){
return this.replace(/[A-Za-z]+://[A-Za-z0-9-_]+.[A-Za-z0-9-_:%&;?/.=]+/g,function(m){
return m.link(m);
});
};
String.prototype.linkuser=function(){
return this.replace(/[@]+[A-Za-z0-9-_]+/g,function(u){
return u.link("http://twitter.com/"+u.replace("@",""));
});
};
String.prototype.linktag=function(){
return this.replace(/[]+[A-Za-z0-9-_]+/,function(t){
return t;
});
};
//load twitter stylesheet
$("head").append('<link rel="stylesheet" type="text/css" href="css/style.css"/>');
//get the tweets from Twitter API
$.getJSON("http://search.twitter.com/search.json?q=jquery4u&rpp=5&callback=?", function(data)
{
// console.log(data.items.length);
$(data.results).each(function(i,v)
{
var tTime=new Date(Date.parse(this.created_at));
var cTime=new Date();
var sinceMin=Math.round((cTime-tTime)/60000);
if(sinceMin==0){
var sinceSec=Math.round((cTime-tTime)/1000);
if(sinceSec<10)
var since='less than 10 seconds ago';
else if(sinceSec<20)
var since='less than 20 seconds ago';
else
var since='half a minute ago';
}
else if(sinceMin==1){
var sinceSec=Math.round((cTime-tTime)/1000);
if(sinceSec==30)
var since='half a minute ago';
else if(sinceSec<60)
var since='less than a minute ago';
else
var since='1 minute ago';
}
else if(sinceMin<45)
var since=sinceMin+' minutes ago';
else if(sinceMin>44&&sinceMin<60)
var since='about 1 hour ago';
else if(sinceMin<1440){
var sinceHr=Math.round(sinceMin/60);
if(sinceHr==1)
var since='about 1 hour ago';
else
var since='about '+sinceHr+' hours ago';
}
else if(sinceMin>1439&&sinceMin<2880)
var since='1 day ago';
else{
var sinceDay=Math.round(sinceMin/1440);
var since=sinceDay+' days ago';
}
var tweetBy='<a class="tweet-user" target="_blank" href="http://twitter.com/'+this.from_user+'">@'+this.from_user+'</a> <span class="tweet-time">'+since+'';
tweetBy=tweetBy+' · <a class="tweet-reply" target="_blank" href="http://twitter.com/?status=@'+this.from_user+' &in_reply_to_status_id='+this.id+'&in_reply_to='+this.from_user+'">Reply</a>';
tweetBy=tweetBy+' · <a class="tweet-view" target="_blank" href="http://twitter.com/'+this.from_user+'/statuses/'+this.id+'">View Tweet</a>';
tweetBy=tweetBy+' · <a class="tweet-rt" target="_blank" href="http://twitter.com/?status=RT @'+this.from_user+' '+escape(this.text.replace(/"/g,'"'))+'&in_reply_to_status_id='+this.id+'&in_reply_to='+this.from_user+'">RT</a>';
var tweet='<div class="tweet"><div class="tweet-left"><a target="_blank" href="http://twitter.com/'+this.from_user+'"><img width="48" height="48" alt="'+this.from_user+' on Twitter" src="'+this.profile_image_url+'" /></a></div><div class="tweet-right"><p class="text">'+this.text.linkify().linkuser().linktag().replace(/<a /g,'<a target="_blank"')+'<br></a>'+tweetBy+'</p></div><br style="clear: both;" /></div>';
$('#twitter').append(tweet); //add the tweet...
});
});
}
}
})(jQuery,window,document);
Some of the code used above is courtesy of TwitStream. Thanks guys.
Frequently Asked Questions (FAQs) about JavaScript Twitter Search API
How can I authenticate my Twitter API requests using JavaScript?
To authenticate your Twitter API requests, you need to use OAuth 2.0. This involves creating a Twitter Developer account, creating an app, and obtaining the necessary keys and tokens. You can then use these keys and tokens in your JavaScript code to authenticate your requests. Here’s a simple example:const Twitter = require('twitter');
let client = new Twitter({
consumer_key: 'your-consumer-key',
consumer_secret: 'your-consumer-secret',
access_token_key: 'your-access-token-key',
access_token_secret: 'your-access-token-secret'
});
How can I use the Twitter Search API to search for tweets containing specific keywords?
The Twitter Search API allows you to search for tweets containing specific keywords. You can use the statuses/user_timeline
endpoint to get the most recent tweets from a specific user. Here’s an example:client.get('statuses/user_timeline', {screen_name: 'username', count: 20}, function(error, tweets, response) {
if (!error) {
console.log(tweets);
}
});
How can I handle errors when using the Twitter Search API?
When using the Twitter Search API, errors can be handled using the standard JavaScript error handling techniques. This typically involves using a try-catch block to catch any errors that occur during the execution of your code. Here’s an example:try {
client.get('statuses/user_timeline', {screen_name: 'username', count: 20}, function(error, tweets, response) {
if (error) throw error;
console.log(tweets);
});
} catch (error) {
console.error('An error occurred:', error);
}
How can I paginate results when using the Twitter Search API?
The Twitter Search API provides a max_id
parameter that you can use to paginate results. This parameter specifies the maximum ID of the tweets you want to retrieve. By setting this parameter to the ID of the last tweet you retrieved, you can get the next set of tweets. Here’s an example:client.get('statuses/user_timeline', {screen_name: 'username', count: 20, max_id: 'last-tweet-id'}, function(error, tweets, response) {
if (!error) {
console.log(tweets);
}
});
How can I filter tweets by language when using the Twitter Search API?
The Twitter Search API provides a lang
parameter that you can use to filter tweets by language. This parameter accepts a language code (like ‘en’ for English, ‘es’ for Spanish, etc.) and returns only tweets in that language. Here’s an example:client.get('statuses/user_timeline', {screen_name: 'username', count: 20, lang: 'en'}, function(error, tweets, response) {
if (!error) {
console.log(tweets);
}
});
How can I include retweets and replies in my search results?
By default, the Twitter Search API does not include retweets and replies in the search results. However, you can include them by setting the include_rts
and exclude_replies
parameters to ‘true’. Here’s an example:client.get('statuses/user_timeline', {screen_name: 'username', count: 20, include_rts: true, exclude_replies: false}, function(error, tweets, response) {
if (!error) {
console.log(tweets);
}
});
How can I limit the number of tweets returned by the Twitter Search API?
The Twitter Search API provides a count
parameter that you can use to limit the number of tweets returned. This parameter accepts a number between 1 and 200, and returns that many tweets. Here’s an example:client.get('statuses/user_timeline', {screen_name: 'username', count: 10}, function(error, tweets, response) {
if (!error) {
console.log(tweets);
}
});
How can I search for tweets from a specific user using the Twitter Search API?
The Twitter Search API provides a from:
operator that you can use to search for tweets from a specific user. This operator accepts a username and returns only tweets from that user. Here’s an example:client.get('search/tweets', {q: 'from:username'}, function(error, tweets, response) {
if (!error) {
console.log(tweets);
}
});
How can I search for tweets containing a specific hashtag using the Twitter Search API?
The Twitter Search API provides a #
operator that you can use to search for tweets containing a specific hashtag. This operator accepts a hashtag and returns only tweets containing that hashtag. Here’s an example:client.get('search/tweets', {q: '#hashtag'}, function(error, tweets, response) {
if (!error) {
console.log(tweets);
}
});
How can I search for tweets containing a specific URL using the Twitter Search API?
The Twitter Search API provides a filter:links
operator that you can use to search for tweets containing a specific URL. This operator accepts a URL and returns only tweets containing that URL. Here’s an example:client.get('search/tweets', {q: 'filter:links url.com'}, function(error, tweets, response) {
if (!error) {
console.log(tweets);
}
});
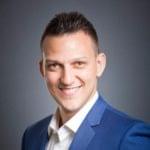
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.