- GPT-4 and Debugging
- Fixing Syntax Errors in Python Code
- Generating Automated Test Cases for Java Programs
- Suggesting Code Optimizations for C++ Programs
- Completing Partial JavaScript Code Snippets
- Checking for Security Vulnerabilities in PHP Code
- Automating Bug Fixing in Ruby on Rails Applications
- Finding and Fixing Memory Leaks in C# Programs
- Automatically Detecting and Correcting SQL Injection Vulnerabilities in Databases
- Completing Code Snippets for Machine Learning Algorithms in Python
- Conclusion
- Frequently Asked Questions (FAQs) about GPT-4 for Debugging
In this tutorial, we’ll explore how to harness the power of GPT-4 to detect bugs in our code and help with fixing them.
We’ll learn how to do the following:
- fix syntax errors in Python code
- generate automated test cases for Java programs
- suggest code optimizations for C++ programs
- complete partial JavaScript code snippets
- check for security vulnerabilities in PHP code
- automate bug fixing in Ruby on Rails applications
- find and fix memory leaks in C# programs
- automatically detect and correct SQL injection vulnerabilities in databases
- and complete code snippets for machine learning algorithms in Python
GPT-4 and Debugging
As web developers, we often find ourselves spending hours debugging our code. Perhaps we were trying to fix a syntax error in our Python code, generating test cases for our Java programs, optimizing our C++ programs, completing partial JavaScript code snippets, checking for security vulnerabilities in our PHP code, automating bug fixing in our Ruby on Rails applications, generating API documentation for our Flask web applications, finding and fixing memory leaks in our C# programs, automatically detecting and correcting SQL injection vulnerabilities in our databases, or completing code snippets for machine learning algorithms in Python.
These situations are often time-consuming and tedious. But we can now leverage GPT-4 for code completion and debugging. GPT-4 is a powerful AI language model that can automate repetitive programming tasks and suggest fixes for errors in code.
Fixing Syntax Errors in Python Code
Python is a popular programming language that’s widely used for web development. One of the most common mistakes that Python programmers make is syntax errors. These errors occur when the code isn’t written correctly and doesn’t follow the syntax rules of the Python language. Syntax errors can be time-consuming to debug and can cause frustration for developers.
To leverage GPT-4 for fixing syntax errors in Python code, the following steps can be followed:
- Identify the line of code that contains the syntax error.
- Copy the line of code into a text editor or IDE.
- Use GPT-4 to generate a corrected version of the line of code.
- Replace the incorrect line of code with the corrected version generated by GPT-4.
- Test the corrected code to ensure that the syntax error has been fixed.
Here’s an example of how this can be accomplished using GPT-4:
# Incorrect code
for i in range(10)
print(i)
# Syntax error: Missing colon at the end of the for loop declaration
# Corrected code generated by GPT-4
for i in range(10):
print(i)
Generating Automated Test Cases for Java Programs
Automated testing is an essential part of software development that saves time and reduces the risk of introducing bugs into code. Java is a popular programming language for creating web applications, and automated testing can be used to ensure that the code behaves as expected and meets the requirements of the project.
To leverage GPT-4 for generating automated test cases for Java programs, the following steps can be followed:
- Identify the Java class or method that needs to be tested.
- Use GPT-4 to generate a test case for the Java class or method.
- Save the test case to a file.
- Run the test case using a testing framework such as JUnit.
- Fix any errors or failures in the test case until it passes.
Here’s an example of how this can be accomplished using GPT-4:
// Java class to be tested
public class Calculator {
public int add(int a, int b) {
return a + b;
}
}
// GPT-4 generated test case
import static org.junit.Assert.*;
import org.junit.Test;
public class CalculatorTest {
@Test
public void testAdd() {
Calculator calculator = new Calculator();
int result = calculator.add(2, 3);
assertEquals(5, result);
}
}
Suggesting Code Optimizations for C++ Programs
C++ is a powerful programming language that’s widely used for systems programming, game development, and other high-performance applications. C++ programmers are always looking for ways to optimize their code to improve performance and reduce memory usage.
To leverage GPT-4 for suggesting code optimizations for C++ programs, the following steps can be followed:
- Identify the section of code that needs to be optimized.
- Use GPT-4 to suggest potential optimizations for the code.
- Implement the suggested optimizations in the code.
- Test the optimized code to ensure that it still functions correctly.
Here’s an example of how this can be accomplished using GPT-4:
// Original C++ code
for (int i = 0; i < n; i++) {
for (int j = 0; j < m; j++) {
a[i][j] = i * j;
}
}
// GPT-4 suggested optimization
for (int i = 0; i < n; i++) {
int temp = i * j;
for (int j = 0; j < m; j++) {
a[i][j] = temp;
}
}
Completing Partial JavaScript Code Snippets
JavaScript is a popular programming language for creating interactive web applications. JavaScript programmers often find themselves in a situation where they need to complete partial code snippets to get their application to function correctly.
To leverage GPT-4 for completing partial JavaScript code snippets, the following steps can be followed:
- Identify the partial JavaScript code snippet that needs to be completed.
- Use GPT-4 to generate the missing code.
- Copy the generated code into the original code snippet.
- Test the completed code to ensure that it works as expected.
Here’s an example of how this can be accomplished using GPT-4:
// Partial JavaScript code snippet
var numbers = [1, 2, 3];
var squares = numbers.map(function(num) {
return;
});
// GPT-4 generated code
var numbers = [1, 2, 3];
var squares = numbers.map(function(num) {
return num * num;
});
Checking for Security Vulnerabilities in PHP Code
PHP is a popular programming language for creating web applications. However, PHP code can be vulnerable to security attacks such as SQL injection, cross-site scripting, and command injection. Developers need to ensure that their PHP code is secure and doesn’t contain any vulnerabilities that could be exploited by attackers.
To leverage GPT-4 for checking for security vulnerabilities in PHP code, the following steps can be followed:
- Identify the PHP code that needs to be checked for security vulnerabilities.
- Use GPT-4 to analyze the code and identify potential vulnerabilities.
- Implement the suggested fixes for any identified vulnerabilities.
- Test the fixed code to ensure that it is secure.
Here’s an example of how this can be accomplished using GPT-4:
// PHP code vulnerable to SQL injection
$id = $_GET['id'];
$sql = "SELECT * FROM users WHERE id='$id'";
// GPT-4 suggested fix
$id = mysqli_real_escape_string($_GET['id']);
$sql = "SELECT * FROM users WHERE id='$id'";
Automating Bug Fixing in Ruby on Rails Applications
Ruby on Rails is a popular web application framework that’s widely used for building web applications. Developers often find themselves in a situation where they need to fix bugs in their Rails apps. Automating bug fixing in Ruby on Rails apps can save time and reduce the risk of introducing new bugs into the code.
To leverage GPT-4 for automating bug fixing in Ruby on Rails applications, the following steps can be followed:
- Identify the bug that needs to be fixed.
- Use GPT-4 to analyze the code and identify potential fixes for the bug.
- Implement the suggested fixes.
- Test the fixed code to ensure that the bug has been fixed.
Here’s an example of how this can be accomplished using GPT-4:
# Ruby on Rails code with a bug
def index
@products = Product.where('name like ?', "%#{params[:search]}%")
end
# GPT-4 suggested fix
def index
@products = Product.where('name like ?', "#{params[:search]}")
end
Finding and Fixing Memory Leaks in C# Programs
C# is a popular programming language for Windows application development. Memory leaks can occur in C# programs when the program allocates memory but doesn’t release it, leading to performance degradation and potentially causing the program to crash.
To leverage GPT-4 for finding and fixing memory leaks in C# programs, the following steps can be followed:
- Identify the section of code that is causing the memory leak.
- Use GPT-4 to analyze the code and identify the source of the memory leak.
- Implement the suggested fix to release the allocated memory.
- Test the fixed code to ensure that the memory leak has been fixed.
Here’s an example of how this can be accomplished using GPT-4:
// C# code with a memory leak
void ReadFile() {
FileStream stream = new FileStream(filename, FileMode.Open);
StreamReader reader = new StreamReader(stream);
string contents = reader.ReadToEnd();
// Forgot to close stream and reader
}
// GPT-4 suggested fix
void ReadFile() {
FileStream stream = new FileStream(filename, FileMode.Open);
StreamReader reader = new StreamReader(stream);
string contents = reader.ReadToEnd();
reader.Close();
stream.Close();
}
Automatically Detecting and Correcting SQL Injection Vulnerabilities in Databases
SQL injection is a common vulnerability in web applications that use databases. Attackers can exploit SQL injection vulnerabilities to execute malicious SQL queries that can steal data or damage the system. Developers need to ensure that their databases are secure and don’t contain any SQL injection vulnerabilities.
To leverage GPT-4 for automatically detecting and correcting SQL injection vulnerabilities in databases, the following steps can be followed:
- Identify the database that needs to be checked for SQL injection vulnerabilities.
- Use GPT-4 to analyze the database and identify potential SQL injection vulnerabilities.
- Implement the suggested fixes for any identified vulnerabilities.
- Test the fixed database to ensure that it is secure.
Here’s an example of how this can be accomplished using GPT-4:
-- SQL code vulnerable to SQL injection
SELECT * FROM users WHERE username = '$username' AND password = '$password'
-- GPT-4 suggested fix
USE account;
SELECT * FROM users WHERE username = ? AND password = ?;
Completing Code Snippets for Machine Learning Algorithms in Python
Python is a popular programming language for creating machine learning applications. Machine learning algorithms can be complex, and developers often need to complete partial code snippets to implement them correctly.
To leverage GPT-4 for completing code snippets for machine learning algorithms in Python, the following steps can be followed:
- Identify the partial code snippet that needs to be completed.
- Use GPT-4 to generate the missing code.
- Copy the generated code into the original code snippet.
- Test the completed code to ensure that it works as expected.
Here’s an example of how this can be accomplished using GPT-4:
# Partial code snippet for a neural network in Python
model = Sequential()
model.add(Dense(32, input_dim=784, activation='relu'))
model.add(Dense(10, activation='softmax'))
# GPT-4 generated code
model = Sequential()
model.add(Dense(32, input_dim=784, activation='relu'))
model.add(Dense(32, activation='relu'))
model.add(Dense(10, activation='softmax'))
# Completes the neural network architecture with an additional hidden layer
Conclusion
In this tutorial, we’ve explored how to leverage GPT-4 for code completion and debugging to automate repetitive programming tasks and suggest fixes for errors in code.
We’ve covered examples of how GPT-4 can be used to fix syntax errors in Python code, generate automated test cases for Java programs, suggest code optimizations for C++ programs, complete partial JavaScript code snippets, check for security vulnerabilities in PHP code, automate bug fixing in Ruby on Rails applications, find and fix memory leaks in C# programs, automatically detect and correct SQL injection vulnerabilities in databases, and complete code snippets for machine learning algorithms in Python.
By using GPT-4, developers can save time and reduce the risk of introducing bugs into their code. GPT-4 is a powerful tool that can help developers become more productive, efficient, and effective in their work.
Frequently Asked Questions (FAQs) about GPT-4 for Debugging
What is GPT-4 and how does it help in debugging?
GPT-4, or Generative Pretrained Transformer 4, is an advanced version of the AI language model developed by OpenAI. It’s designed to understand and generate human-like text based on the input it receives. In the context of debugging, GPT-4 can be a valuable tool. It can analyze code, identify potential errors, and suggest corrections. This can significantly reduce the time and effort developers spend on debugging, making the process more efficient.
How does GPT-4 differ from its predecessor, GPT-3?
While both GPT-3 and GPT-4 are AI language models developed by OpenAI, GPT-4 is a more advanced version. It has a larger model size, which means it can process and understand more complex language patterns. This makes it more accurate and efficient in tasks like debugging, where understanding complex code structures is crucial.
Can GPT-4 replace human developers in debugging?
While GPT-4 is a powerful tool for debugging, it’s not designed to replace human developers. It can identify and suggest corrections for code errors, but it still requires a human to review and implement these suggestions. GPT-4 is a tool that can assist developers, making their work more efficient, but it doesn’t eliminate the need for human expertise and judgment.
How can I integrate GPT-4 into my debugging process?
Integrating GPT-4 into your debugging process requires some technical knowledge. You’ll need to install the necessary software and configure it to work with your codebase. This might involve setting up an API connection to the GPT-4 service, or installing a plugin or extension if you’re using a supported IDE.
Is GPT-4 suitable for all types of programming languages?
GPT-4 is a versatile tool that can work with a variety of programming languages. However, its effectiveness might vary depending on the complexity and structure of the language. It’s always a good idea to test GPT-4 with your specific language and codebase to see how well it performs.
What are the limitations of using GPT-4 for debugging?
While GPT-4 is a powerful tool, it’s not perfect. It might not always correctly identify or fix all errors in your code. It also requires a certain level of technical knowledge to set up and use effectively. Additionally, like all AI models, it’s only as good as the data it’s trained on, so it might not perform as well with unfamiliar or highly specialized code.
How does GPT-4 handle complex debugging tasks?
GPT-4 is designed to handle complex language patterns, which makes it well-suited for complex debugging tasks. It can analyze intricate code structures, identify potential errors, and suggest corrections. However, the accuracy and effectiveness of these suggestions can vary, and they should always be reviewed by a human developer.
Can GPT-4 help with debugging in real-time?
Yes, GPT-4 can be used for real-time debugging. It can analyze code as it’s being written, identify potential errors, and suggest corrections on the fly. This can help developers catch and fix errors more quickly, improving the efficiency of the coding process.
Is GPT-4 secure to use for debugging?
As an AI model developed by OpenAI, GPT-4 is designed with security in mind. However, like any tool, it’s important to use it responsibly. This includes ensuring that your code and data are secure when using GPT-4, and reviewing any suggestions it makes before implementing them.
What is the future of using AI like GPT-4 in debugging?
The use of AI in debugging is a rapidly evolving field. Tools like GPT-4 are becoming more advanced and capable, and we can expect them to play an increasingly important role in the debugging process. However, they’re not likely to replace human developers entirely. Instead, they’ll serve as valuable tools that can make the debugging process more efficient and effective.
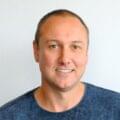
Mark Harbottle is the co-founder of SitePoint, 99designs, and Flippa.