WordPress has supported the Transients API since version 2.8 but still many WordPress developers are unaware of its existence and uses. In a nutshell, WordPress Transients API lets us store key-value pairs of data with an expiration time.
In this tutorial, we will cover how to use this API in depth. We will also see how it’s different from Options API, how it interacts with the WordPress caching system and few of its use cases.
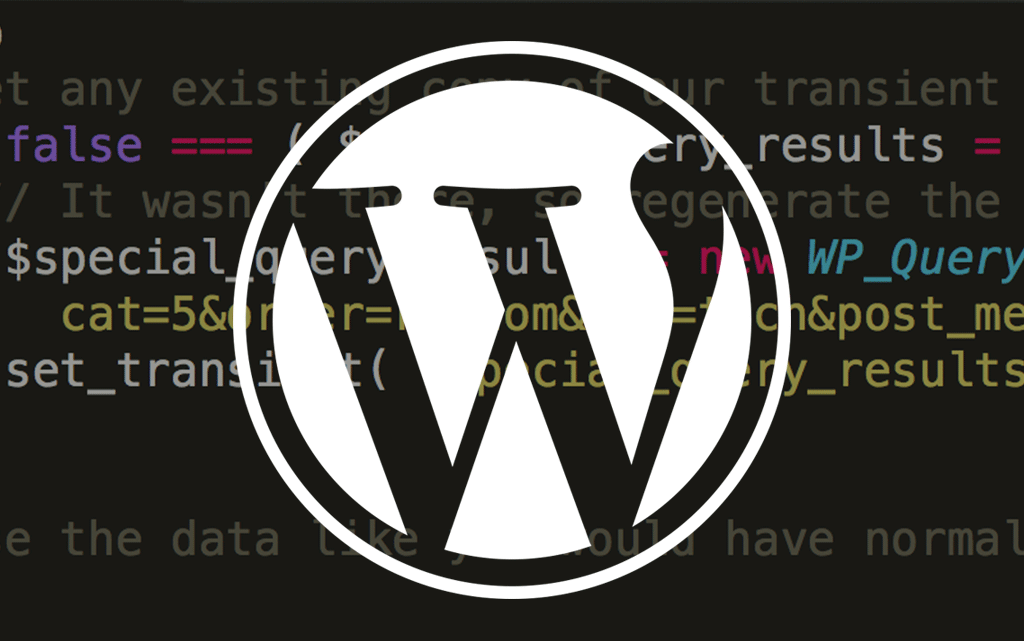
Options API vs Transients API
Most WordPress developers are aware of WordPress Options API. Options API lets us store key-value pairs of data permanently in the database. What many WordPress developers don’t realise is that Options API implements a caching layer (i.e. WordPress Object Cache) to cache the options. If a persistent cache is not enabled then a new caching session is created on every HTTP request otherwise the persistent cache is used by Options API.
Almost every WordPress API interacts with MySQL using the WordPress Object Cache to cache the data to prevent multiple MySQL hits.
Transients API works a little different than all the other APIs. It stores the key-value pairs of data in MySQL only if a persistent cache is not enabled otherwise it uses the Object Cache only. Whereas all other APIs use both to synchronise the data to make sure the data is persistent. Therefore Transients are not persistent i.e. shouldn’t be used to store critical data. So Transients API is great for caching data.
Note: If a persistent cache is not enabled then Transients API uses Options API to store the key-value pairs of data otherwise it uses the Object Cache directly. Transients are stored in Options table. Every transient is made up of two options i.e. key-value pair data and key-value pair expiration date.
Create a Transient
To set a transient we need to use set_transient()
function. This function takes three parameters:
- Transient name(required): Must be a string. Length of the string cannot be more than 40 characters otherwise it will fail to create the transient.
- Transient value(required): Must be a string. If you pass a object or array then it’s serialized i.e. converted to a string.
- Expiration Seconds(optional): Seconds within which the transient will expire in. Transient may also expire before the expiration time because cached data (i.e. data stored in Object cache) is volatile.
Here is an example of code using set_transient()
function:
set_transient("Website", "SitePoint", 3600);
Here we are storing a key named ‘Website’ with value ‘SitePoint’ for 1 hour. After 1 hour the key will not be accessible anymore.
set_transient
returns true if the transient is created successfully otherwise it returns false.
If you don’t provide an expiration time or provide ‘0’ as the expiration time then it never expires the transient.
Note: If expiration time is not provided or if expiration time is ‘0’ then transients are autoloaded (i.e. loaded into memory whenever a page is requested).
Transients API also provides another function to create a transient i.e. set_site_transient
. It also takes the same three parameters like set_transient
. Most of the functionality between them is the same. The differences between set_transient
and set_site_transient
are:
- When
set_site_transient
is used with a Multi Site network then the transient is available for all the sites in the network. - Whether there is a expiration time or not, transients created using
set_site_transient
are always auto loaded.
Finally, if you run set_transient
of an existing transient key, then the value and expiration time is updated with the newly provided value and expire time. The expire time is calculated from the first time the transient was set.
Retrieving a Transient
To retrieve a stored transient you need to use get_transient
function. It just takes one parameter i.e. the name of the transient.
$value = get_transient("Website");
if($value === false)
{
echo "Expired or not found";
}
get_transient
returns false if the transient is expired or is not present. Otherwise it returns the value of the transient.
A transient will return false if it’s expired or not found, therefore you should never store boolean values in a transient. If you want to store boolean values then use the integers form i.e 0 or 1.
If you have set a transient using set_site_transient
then use get_site_transient
to retrieve it instead of get_transient
.
Deleting a Transient
To delete a transient you need to use delete_transient
function. It takes only one parameter i.e. the name of the transient.
Here’s an example:
delete_transient("Website");
It returns true if the transient was deleted successfully. If the transient is not found or due to another reason, it can’t delete the transient and then it returns false.
If you have set a transient using set_site_transient
then use delete_site_transient
to delete it instead of delete_transient
.
Retrieving and Caching Posts of a Particular Category
The transients API can be used to cache anything. Most plugins use this API to cache data. For the sake of an example, let’s see how to retrieve and cache posts of a category.
<?php
if(($posts = get_transient("sitepoint_wordpress_posts")) === false)
{
$posts = new WP_Query("category_name=wordpress");
set_transient("sitepoint_wordpress_posts", $posts, 3600);
}
?>
<?php if($posts->have_posts()): ?>
<?php while($posts->have_posts()) : $posts->the_post(); ?>
//display the post
<?php endwhile; ?>
<?php else: ?>
//display no posts found message
<?php endif; ?>
<?php wp_reset_postdata(); ?>
Here we are caching the category posts for an hour. We are retrieving the posts using WP_Query
class. WP_Query
is serialized and stored as a transient. While retrieving it gets deserialized.
Final Thoughts
This article demonstrates how easily we can cache data in WordPress using the Transients API.
You can enable a persistent cache in WordPress using either Memcached Object Cache or the WP Redis plugins.
Please let me know your experiences with this API in the comments below.
Frequently Asked Questions (FAQs) about WordPress Transients API
What is the main purpose of using WordPress Transients API?
The primary purpose of using the WordPress Transients API is to store temporary data that can help speed up your WordPress site. It allows developers to store data with an expiration time. This data could be anything from the results of a complex database query to simple string values. By storing this data, your WordPress site can retrieve it quickly without needing to regenerate it every time, thus improving the site’s performance.
How does the WordPress Transients API improve website performance?
The WordPress Transients API improves website performance by reducing the number of database queries. When data is stored using the Transients API, it can be quickly retrieved from the cache instead of executing a new database query. This significantly reduces the load on the server and speeds up the page load time, providing a better user experience.
Can I use WordPress Transients API for permanent data storage?
No, the WordPress Transients API is not designed for permanent data storage. The data stored using the Transients API is temporary and has an expiration time. Once the data expires, it is automatically deleted from the cache. If you need to store data permanently, you should use other WordPress APIs like the Options API.
How can I delete a transient in WordPress?
You can delete a transient in WordPress using the delete_transient function. This function takes the name of the transient as its argument. Here’s an example:delete_transient( 'my_transient' );
In this example, ‘my_transient’ is the name of the transient you want to delete.
What happens if I try to retrieve an expired transient?
If you try to retrieve an expired transient, the WordPress Transients API will return false. This is because the data is automatically deleted from the cache once it expires. You should always check if the transient is still valid before trying to use the data.
Can I set a transient to never expire?
While you can technically set a transient to never expire by giving it a very long expiration time, it’s not recommended. Transients are meant for temporary data storage, and setting them to never expire could lead to unnecessary data buildup in your cache.
How can I manage and delete transients in WordPress?
You can manage and delete transients in WordPress using various plugins like Transient Manager, WP-Optimize, and Transients Manager. These plugins provide a user-friendly interface to view, delete, and manage all your transients.
Can I use WordPress Transients API on a multisite installation?
Yes, you can use the WordPress Transients API on a multisite installation. However, you should use the set_site_transient and get_site_transient functions instead of set_transient and get_transient. These functions work across the entire network of sites.
What is the difference between a transient and a cookie?
A transient and a cookie serve different purposes. A transient is used to store temporary data on the server side to improve website performance. On the other hand, a cookie is used to store data on the client side, usually to remember user preferences and sessions.
Can I store arrays or objects using the WordPress Transients API?
Yes, you can store arrays or objects using the WordPress Transients API. The API automatically serializes these data types for you, so you can store them as transients and retrieve them later without any issues.

Narayan is a web astronaut. He is the founder of QNimate. He loves teaching. He loves to share ideas. When not coding he enjoys playing football. You will often find him at QScutter classes.