In this article, we’ll introduce .NET Blazor, a powerful framework that unifies client-side and server-side development paradigms and offers enhanced performance and improved tooling.
Introducing .NET Blazor
One of the main challenges developers face often is that they need to know two different languages — one for the server-side development, and one for the client-side development. .NET Blazor tries to bridge the gap between client-side and server-side development by enabling developers to build interactive web applications using C# and .NET. So developers can rely on a single development language framework and reuse the experience and knowledge they already have.
This issue was the main reason behind Microsoft’s .NET Blazor framework. It actually started as a personal side-project of Steven Sanderson, Principal Software Engineering Lead at Microsoft in 2017, which evolved into server-side Blazor mid 2019, and client-side (WebAssembly) in 2020.
In this article, we’ll start from the recent history of web app development, and the main differences between server-side and client-side web app architectures. From there, we’ll map both architectures with current corresponding Blazor hosting models. In the second part of the article, we’ll learn about the upcoming changes in the next Blazor release based on .NET 8 later in 2023, and how to develop our first Blazor app using the current .NET 8 preview 7.
The Recent History of Web App Development
The world of web app development can be summarized in server-side and client-side architectures.
Server-side, as the name implies, requires an underlying web server, such as Windows Internet Information Server (IIS), Linux Apache, or NGINX, or a containerized platform version of the same.
Server-side development relies on generating HTML on the server and sending it to the client. Technologies like ASP.NET Web Forms provide rich controls and abstractions, making it easier to build complex web applications. However, the tight coupling between server and client often leads to challenges in maintaining scalability and responsiveness. If the client can’t reach the server, there’s no web page (or a page saying HTTP 404 error). Various languages are used for the actual web application development, such as C# .NET, Java, and PHP.
Client-side refers to a web application scenario, which doesn’t require a server in the backend, but rather runs completely in the browser.
The evolution of web app development began with static HTML pages. As the demand for dynamic content and interactivity grew, technologies like JavaScript emerged, enabling developers to build more interactive web applications. The early 2000s witnessed the rise of AJAX (Asynchronous JavaScript and XML), which allowed for asynchronous data exchange between the client and the server, resulting in smoother user experiences. Frameworks like jQuery further simplified client-side scripting. Two decades later, the websites we’re visiting daily are still primarily based on HTML and JavaScript.
Blazor Hosting Models
.NET Blazor offers two main hosting models: Blazor Server and Blazor WebAssembly.
Blazor Server allows developers to create rich and dynamic web applications, where the user interface logic is 100% executed on the server, and the UI updates get sent to the client over a persistent SignalR connection. This model provides real-time interactivity while maintaining a familiar programming model for .NET developers. It’s well suited for applications that require dynamic updates such as pulling up database information in a retail/eshop scenario, customer profiles, financial stock reporting and the like, as well as typically having a lower latency tolerance.
The image below presents a diagram of Blazor server architecture.
Let’s look at some of the pros and cons of server-side Blazor.
Pros:
- Fast load time, assuming the web server is sized correctly.
- Closest to traditional ASP.NET Core development.
- Support for older browsers, as no requirement for WebAssembly (although this could be seen as a negative point from a security and supportability perspective).
Cons:
- Client/Browser consumes more memory to run the web app, and is 100% dependent on the SignalR connection.
- Each client session consumes CPU and memory on the server, which might bring in right-size challenges for applications under heavy or spike load.
- Client <-> Server communication assumes a “strong” connection to avoid latency and errors.
Blazor WebAssembly takes a totally different approach, allowing developers to run .NET code directly in the browser using WebAssembly (aka Wasm), a binary instruction format for web applications. This model allows Blazor to run the execution of C# code on the client side, reducing the need for constant communication with the server. Blazor WebAssembly is ideal for scenarios where applications need to be fully client-side, yet still providing a rich and responsive user experience — similar to what users typically expect from server applications.
The image below presents a diagram of Blazor WebAssembly architecture.
Let’s look at some of the pros and cons of WebAssembly.
Pros:
- A website can be deployed as static files, since it all runs in the browser.
- Wasm Apps can run offline, as there is no need for server connection (all the time).
- Support for progressive web apps (PWAs), which means it can act as a locally installed application on the client machine.
Cons:
- Since the JavaScript engine in the browser needs to download the full Blazor app and corresponding .NET DLLs, the first load of the app could be considered relatively slow.
- WebAssembly requires a modern browser. If old browsers are still in use and required, you can only use Blazor Server.
- If installed as a PWA, there’s a challenge around version updates and management.
- Code and DLLs can be decrypted. Any secrets such as connection strings, passwords and the like shouldn’t be used within the code, as they’re visible in the browser dev tools.
Note: Blazor has two other hosting models — Hybrid, which targets desktops and mobile platforms, and Mobile Blazor Bindings, which is experimental and aiming for multi-platform scenarios such as iOS and Android, besides Windows and macOS.
No matter the runtime version, Blazor apps are created using Razor Components, sometimes also known as Blazor Components or just components. Each component is a stand-alone piece of a UI-element, typically formed by a combination of HTML code for the page layout, and a snippet of C# code for the logic and dynamic content.
The code below shows a sample Blazor component, with a counter field and a button in the @page(HTML)
section, where the logic is in the @code
section:
@page "/counter"
<h1>Counter</h1>
<p>Current count: @currentCount</p>
<button class="btn btn-primary" @onclick="IncrementCount">Click me</button>
@code {
private int currentCount = 0;
private void IncrementCount()
{
currentCount++;
}
}
The future of Blazor with .NET 8
In November 2023, Microsoft will release the .NET 8 framework, which is currently in preview v7 (see the .Net team’s announcements here).
Specifically for Blazor, the following changes are on the current roadmap.
Server-side Rendering
Server-side rendering follows the current logic of Razor pages or MVC Applications, similar to ASP.NET Web Forms previously. Razor Pages is a page-based model. UI and business logic concerns are kept separate, but within the page.
Razor Pages is the recommended way to create new page-based or form-based apps for developers new to ASP.NET Core. It provides an easier starting point than ASP.NET Core MVC. ASP.NET MVC renders UI on the server and uses a model-view-controller (MVC) architectural pattern.
The MVC pattern separates an app into three main groups of components: models, views, and controllers. User requests are routed to a controller. The controller is responsible for working with the model to perform user actions or retrieve results of queries. The controller chooses the view to display to the user, and provides it with any model data it requires. Support for Razor Pages is built on ASP.NET Core MVC.
Thanks to server-side rendering (SSR), the server generates the HTML code in response to a request from the browser/client. The big benefit with SSR is that performance will dramatically increase, as there’s no WebAssembly object to be downloaded when loading the app. Compared with using Razor pages or MVC — which technically does the same as what we explained in the previous sentence — developers can benefit from Blazor’s component-based architecture, which doesn’t really exist with Razor or MVC. While the component-based approach might feel different at first, once we get the hang of it, we see that a lot of code duplication from the past can now be moved into a reusable Blazor component. Think of buttons, banners, forms, tables,and so on — where the object remains but the data content changes.
Four models in one
Four models in one feels like the ultimate development solution. The current models (server-side, Wasm, hybrid and mobile bindings) are combined with .NET 8 into a single approach, no matter the scenario. Thanks to Blazor, it’s possible to develop rich server-based applications, and client-only apps with Wasm, as well as cross-platform iOS, Android and macOS apps — all based on the same Blazor framework.
Streaming rendering
Streaming rendering is another promising capability in .NET 8 Blazor, which is the middle ground between server-side and client-side rendering. Remember that, with server-side, the full HTML page is rendered by the server. Streaming rendering allows the rendering of the static HTML, as well as placeholders for content. Once the async server-side call is complete — meaning it can stream the data — the actual HTML page is updated by filling in the placeholder objects with the actual data.
Server Side and WebAssembly
Server Side and WebAssembly will still be available in the same way they work with the current version of Blazor, but they’ll be more optimized.
Auto mode
Auto mode is the one I’m personally having most expectations of, and representing the “ultimate” Blazor scenario, allowing a combination of both server-side and WebAssembly in one.
This scenario offers the initial page from the server, which means it will load fast. Subsequently, the necessary objects are downloaded to the client, so the next time the page loads, it’s offered from Wasm. (This version isn’t present in the .NET 8 preview 7 yet, so there aren’t more details to share at the time of writing this.) If you think this is quite similar to the “four models in one” approach described earlier, know that auto mode is targeting browser apps, not full desktop or mobile platform scenarios.
Building Our First Blazor .NET 8 Web App
Now we have a much better understanding of what Blazor is all about, and what’s getting prepared for the next release of .NET 8, let’s walk through a hands-on scenario, where we’ll create our first .NET 8 Blazor app.
Prerequisites
To make this as platform independent as possible, the assumption is you have a machine with the following components:
- Download .NET 8 Preview 7 from here and install it on your development workstation.
- Download Visual Studio Code from here and install it on your development workstation.
- Create a subfolder named
Blazor8sample
. This folder will host the .NET application.
Building the app
With the prerequisites out of the way, let’s walk through the next steps: creating an app using the Blazor template, and running it on our development workstation.
-
Open a terminal window, and browse to the
Blazor8sample
folder created earlier. -
Run the following command to validate the .NET version:
dotnet --version
-
Run the following command to get the details for the new Blazor template:
dotnet new Blazor /?
-
Run the following command to create the new Blazor App:
dotnet new Blazor -o Blazor8SampleApp
-
Navigate to the subfolder
Blazor8SampleApp
, and runcode .
to open the folder in Visual Studio Code. -
From within Visual Studio Code, navigate to Terminal in the top menu, and select New Terminal (or use Ctrl + Shift + ` shortcut keys on Windows). This opens a new Terminal window within VS Code.
-
In the Terminal window, run the following command to launch the Blazor App:
dotnet run .\Blazor8SampleApp
-
Navigate to the localhost listening URL (such as
http://localhost:5211
in my example). Note: the port might be different on your machine, so check the URL for the exact port number.This opens the running Blazor Web App in your default browser. Open the Weather page.
-
From within VS Code, navigate to the
Program.cs
file. The Blazor Web App template is set up for Server-side rendering of Razor components by default. InProgram.cs
the call toAddRazorComponents()
adds the related services, and thenMapRazorComponents<App>()
discovers the available components and specifies the root component for the app. -
When you selected the Weather menu option, the page will have briefly showed Loading, after which it rendered the weather data in a table. This is an example of the Stream Rendering feature as discussed earlier.
-
From within VS Code, navigate to the
/Pages/Weather.razor
page. Open the code view.Notice line 2:
@attribute [StreamRendering(true)]
This allows for the new Blazor Stream Rendering feature to work.
-
Stop the running app by closing the browser, or press Ctrl + C from the terminal window. Update the previous code section to this:
@attribute [StreamRendering(false)]
-
Save the changes, and run the app again by initiating
dotnet run .\ Blazor8SampleApp.csproj
from the terminal window. -
Browse to the running application again by clicking on the
http://localhost:5211
URL, and click the Weather app. Notice how, this time, there’s no Loading… message shown, but it takes a few seconds for the page to render and show the actual weather table.
Summary
The journey of web application development has evolved from static HTML to the dynamic and interactive experiences we enjoy (and expect!) today. With .NET Blazor, Microsoft has taken a significant step in offering developers a powerful framework that unifies client-side and server-side development paradigms.
As we eagerly anticipate the release of .NET Blazor 8, we can look forward to enhanced performance, improved tooling, and features such as server-side as well as stream rendering, which will continue to elevate the web development landscape. Whether you’re a seasoned .NET developer or a newcomer to the ecosystem, .NET Blazor opens doors to building next-generation web applications with the power of C# and .NET.
FAQs About .NET Blazor
Blazor is an open-source web framework developed by Microsoft that allows developers to build interactive web applications using C# and .NET instead of JavaScript. It enables full-stack development by running C# code directly in the browser, making it a powerful alternative to traditional JavaScript frameworks.
Blazor consists of two primary hosting models: Blazor WebAssembly and Blazor Server. Blazor WebAssembly runs C# code in the browser, while Blazor Server runs C# code on the server and uses SignalR to communicate with the client.
Blazor WebAssembly is a client-side hosting model in which the entire Blazor application is downloaded and executed in the user’s browser. This allows Blazor applications to work offline, but it may have slightly slower initial load times compared to Blazor Server.
Blazor Server is a server-side hosting model where the C# code runs on the server and communicates with the client using SignalR. It offers real-time capabilities and is suitable for applications that require server-side processing and minimal client resources.
Yes, Blazor WebAssembly is well-suited for building PWAs. It allows you to create web applications that can be installed on a user’s device and work offline.
Blazor primarily uses C# for writing server-side and client-side code. It also supports Razor, a syntax for building dynamic web pages using C# or HTML. Razor components are a key part of Blazor development.
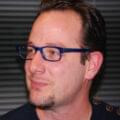
Peter has an extensive background in architecting, deploying, managing and training on Microsoft technologies, dating back to Windows NT4 Server in 1996, all the way to the latest and modern cloud solutions available in Azure today. With a passion for cloud Architecture, Devops and Security, Peter always has a story to share on how to optimize your enterprise-ready cloud workloads. When he's not providing a technical Azure workshop in his role as Azure Technical Trainer at Microsoft Corp (for which he relocated from Belgium to Olympia, Washington, in early 2022), he's developing some new apps using .NET Blazor as a new hobby. Peter was an Azure MVP for five years, has been a Microsoft Certified Trainer (MCT) for over a decade, and is still actively involved in the community as a public speaker, technical writer, book author and publisher.