Helper JS function which sets the price on a specified table cell based on the table id, row id and column number.
function setRowPrice(tableId, rowId, colNum, newValue)
{
$('#'+table).find('tr#'+rowId).find('td:eq(colNum)').html(newValue);
};
Frequently Asked Questions (FAQs) about jQuery and Table Cells
How can I use jQuery to set a specific table cell value?
To set a specific table cell value using jQuery, you need to first select the table cell using the jQuery selector. Once you have selected the cell, you can use the .html() or .text() method to set the value. Here’s an example:$("table tr:nth-child(2) td:nth-child(3)").html("New Value");
In this example, the code is targeting the second row (tr:nth-child(2)) and the third cell in that row (td:nth-child(3)) of the table, and setting its value to “New Value”.
How can I get the value of a specific table cell using jQuery?
To get the value of a specific table cell, you can use the .text() or .html() method after selecting the cell. Here’s an example:var cellValue = $("table tr:nth-child(2) td:nth-child(3)").text();
In this example, the code is getting the value of the third cell in the second row of the table.
Can I change the value of all cells in a specific column using jQuery?
Yes, you can change the value of all cells in a specific column using jQuery. You can do this by selecting all the cells in the column using the :nth-child() selector and then setting their value using the .html() or .text() method. Here’s an example:$("table tr td:nth-child(3)").html("New Value");
In this example, the code is setting the value of all cells in the third column to “New Value”.
How can I use jQuery to add a class to a specific table cell?
You can add a class to a specific table cell using the .addClass() method in jQuery. Here’s an example:$("table tr:nth-child(2) td:nth-child(3)").addClass("myClass");
In this example, the code is adding the class “myClass” to the third cell in the second row of the table.
Can I use jQuery to remove a class from a specific table cell?
Yes, you can remove a class from a specific table cell using the .removeClass() method in jQuery. Here’s an example:$("table tr:nth-child(2) td:nth-child(3)").removeClass("myClass");
In this example, the code is removing the class “myClass” from the third cell in the second row of the table.
How can I use jQuery to change the background color of a specific table cell?
You can change the background color of a specific table cell using the .css() method in jQuery. Here’s an example:$("table tr:nth-child(2) td:nth-child(3)").css("background-color", "yellow");
In this example, the code is changing the background color of the third cell in the second row of the table to yellow.
Can I use jQuery to add a row to a table?
Yes, you can add a row to a table using the .append() method in jQuery. Here’s an example:$("table").append("<tr><td>New Cell</td></tr>");
In this example, the code is adding a new row with one cell to the table.
How can I use jQuery to remove a row from a table?
You can remove a row from a table using the .remove() method in jQuery. Here’s an example:$("table tr:nth-child(2)").remove();
In this example, the code is removing the second row from the table.
Can I use jQuery to add a cell to a specific row in a table?
Yes, you can add a cell to a specific row in a table using the .append() method in jQuery. Here’s an example:$("table tr:nth-child(2)").append("<td>New Cell</td>");
In this example, the code is adding a new cell to the second row of the table.
How can I use jQuery to remove a cell from a specific row in a table?
You can remove a cell from a specific row in a table using the .remove() method in jQuery. Here’s an example:$("table tr:nth-child(2) td:nth-child(3)").remove();
In this example, the code is removing the third cell from the second row of the table.
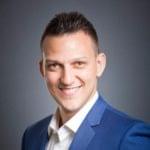
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.