This is a code snippet for basic JavaScript to validate email address using a regular expression. This is building on my previous post on how to use regular expressions with jQuery. You can also load the code in jsfiddle below. Update 12/05/13: Separated into versions for testing.
Version 1
var $email = $('form input[name="email'); //change form to id or containment selector
var re = /^(([^<>()[]\.,;:s@"]+(.[^<>()[]\.,;:s@"]+)*)|(
".+"))@(([[0-9]{1,3}.[0-9]{1,3}.[0-9]{1,3}.[0-9]{1,3}])|(([a-zA
-Z-0-9]+.)+[a-zA-Z]{2,}))$/igm;
if ($email.val() == '' || !re.test($email.val()))
{
alert('Please enter a valid email address.');
return false;
}
Load code in jsfiddle
Version 2
var $email = $('form input[name="email'); //change form to id or containment selector
var re = /[A-Z0-9._%+-]+@[A-Z0-9.-]+.[A-Z]{2,4}/igm;
if ($email.val() == '' || !re.test($email.val()))
{
alert('Please enter a valid email address.');
return false;
}
Load code in jsfiddle
Versions
//reported to validate incorrectly: t123@.co.in as true
/[A-Z0-9._%+-]+@[A-Z0-9-]+.+.[A-Z]{2,4}/igm
//reported to validate incorrectly: andre@uol.com@ as true
/[A-Z0-9._%+-]+@[A-Z0-9.-]+.[A-Z]{2,4}/igm
//current version
/^(([^<>()[]\.,;:s@"]+(.[^<>()[]\.,;:s@"]+)*)|(
".+"))@(([[0-9]{1,3}.[0-9]{1,3}.[0-9]{1,3}.[0-9]{1,3}])|(([a-zA
-Z-0-9]+.)+[a-zA-Z]{2,}))$/
Frequently Asked Questions on JavaScript Email Validation with Regex
Why is it important to validate email addresses in JavaScript?
Email validation is a crucial step in ensuring data integrity and improving user experience in any web application. By validating email addresses, you can prevent users from entering incorrect or fake email addresses, which can lead to communication issues or data inaccuracies. JavaScript, being a client-side language, allows for immediate feedback to the user, making the process more efficient and user-friendly.
What is Regex and how does it work in email validation?
Regex, short for Regular Expression, is a sequence of characters that forms a search pattern. In email validation, Regex is used to match the input with a pattern that corresponds to the standard format of an email address. If the input matches the pattern, it is considered valid; otherwise, it is invalid.
How can I create a simple email validation using Regex in JavaScript?
Here’s a simple example of how you can create an email validation using Regex in JavaScript:function validateEmail(email) {
var re = /^(([^<>()\[\]\\.,;:\s@"]+(\.[^<>()\[\]\\.,;:\s@"]+)*)|(".+"))@((\[[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\])|(([a-zA-Z\-0-9]+\.)+[a-zA-Z]{2,}))$/;
return re.test(String(email).toLowerCase());
}
Why does the Regex pattern for email validation seem so complicated?
The Regex pattern for email validation may seem complicated because it needs to account for the various formats an email address can have. It checks for things like the presence of ‘@’ symbol, the correct sequence of domain name, and the appropriate use of periods and other special characters.
Can Regex be used to validate other forms of input?
Yes, Regex is a versatile tool that can be used to validate various forms of input. It can be used to check if a string contains only numbers, if a password meets certain criteria, if a date is in the correct format, and much more.
What are the limitations of using Regex for email validation in JavaScript?
While Regex is a powerful tool for pattern matching, it has its limitations. For instance, it can’t check if an email address actually exists or if it’s able to receive emails. It can only check if the input matches the standard format of an email address.
How can I improve the user experience when validating email addresses?
One way to improve user experience is to provide immediate feedback. With JavaScript, you can display a message right after the user enters an email address, indicating whether it’s valid or not. This saves the user from having to submit the form to find out if they made a mistake.
Can I customize the Regex pattern for email validation?
Yes, you can customize the Regex pattern to suit your specific needs. However, it’s important to be careful when doing so, as making the pattern too strict might reject valid email addresses, while making it too loose might accept invalid ones.
How can I handle errors in email validation?
You can handle errors by providing clear and helpful error messages. For instance, if the email address is invalid, you can display a message explaining why it’s invalid and how to correct it.
Are there any libraries or tools that can help with email validation in JavaScript?
Yes, there are several libraries and tools available that can simplify email validation in JavaScript. Some popular ones include validator.js, email-validator, and verify.js. These tools provide pre-built functions for email validation, saving you the trouble of writing your own Regex patterns.
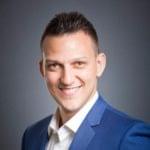
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.