Nowadays, jQuery is far the most favorite Javascript framework of many developers. Through jQuery they will be able to create stunning visual effects, manipulate data, etc. You had been probably in my other posts before so I won’t explain much from what are the benefits can be gained from jQuery.
1. Quick Copy Paste
$(document).ready(function(){
// jQuery Code Here
});
2. Date of Birth
$("#lda-form").submit(function(){
var day = $("#day").val();
var month = $("#month").val();
var year = $("#year").val();
var age = 18;
var mydate = new Date();
mydate.setFullYear(year, month-1, day);
var currdate = new Date();
currdate.setFullYear(currdate.getFullYear() - age);
if ((currdate - mydate) < 0){
alert("Sorry, only persons over the age of " + age + " may enter this site");
return false;
}
return true;
});
3. Text Search
$.fn.egrep = function(pat) {
var out = [];
var textNodes = function(n) {
if (n.nodeType == Node.TEXT_NODE) {
var t = typeof pat == 'string' ?
n.nodeValue.indexOf(pat) != -1 :
pat.test(n.nodeValue);
if (t) {
out.push(n.parentNode);
}
}
else {
$.each(n.childNodes, function(a, b) {
textNodes(b);
});
}
};
this.each(function() {
textNodes(this);
});
return out;
};
$.fn.egrep = function(pat) {
var out = [];
var textNodes = function(n) {
if (n.nodeType == Node.TEXT_NODE) {
var t = typeof pat == 'string' ?
n.nodeValue.indexOf(pat) != -1 :
pat.test(n.nodeValue);
if (t) {
out.push(n.parentNode);
}
}
else {
$.each(n.childNodes, function(a, b) {
textNodes(b);
});
}
};
this.each(function() {
textNodes(this);
});
return out;
};
4. XML file Parser
function parseXml(xml) {
//find every Tutorial and print the author
$(xml).find("Tutorial").each(function()
{
$("#output").append($(this).attr("author") + "");
});
}
5. Class Hover add and Remove
$('.onhover').hover(
function(){ $(this).addClass('hover_style_class') },
function(){ $(this).removeClass('hover_style_class') }
)
6. Partial Page Refresh
setInterval(function() {
$("#refresh").load(location.href+" #refresh>*","");
}, 10000); // seconds to wait, miliseconds
setInterval(function() {
$("#refresh").load(location.href+" #refresh>*","");
}, 10000); // seconds to wait, miliseconds
7. Mouse Position
function rPosition(elementID, mouseX, mouseY) {
var offset = $('#'+elementID).offset();
var x = mouseX - offset.left;
var y = mouseY - offset.top;
return {'x': x, 'y': y};
}
8. Delay Animation or Effect
$(".alert").delay(2000).fadeOut();
9. Popup Windows Opener
jQuery('a.popup').live('click', function(){
newwindow=window.open($(this).attr('href'),'','height=200,width=150');
if (window.focus) {newwindow.focus()}
return false;
});
10. Each Element
$("input").each(function (i) {
//do something with each and pass i as the number of the element
});
Frequently Asked Questions about jQuery Snippets
What are jQuery Snippets and why are they important?
jQuery Snippets are small pieces of reusable code that are used to accomplish specific tasks in jQuery, a popular JavaScript library. They are important because they save developers time and effort by providing ready-made solutions to common programming tasks. These snippets can be easily integrated into larger projects, making them a valuable resource for both novice and experienced developers.
How can I use jQuery Snippets in my projects?
To use a jQuery snippet, you simply need to copy the code and paste it into your project where you want the functionality to be. You may need to modify the code slightly to fit your specific needs, such as changing variable names or adjusting parameters. Always make sure to test the snippet thoroughly in your project to ensure it works as expected.
Are there any prerequisites for using jQuery Snippets?
Yes, to use jQuery snippets, you need to have a basic understanding of JavaScript and jQuery. You also need to include the jQuery library in your project before you can use any jQuery snippets. This can be done by downloading the library from the jQuery website and linking to it in your HTML file, or by linking to a hosted version of the library on a Content Delivery Network (CDN).
Can I create my own jQuery Snippets?
Absolutely! Creating your own jQuery snippets is a great way to improve your coding skills and create custom solutions for your projects. To create a snippet, simply write the jQuery code that accomplishes the task you want, and save it in a separate file or document for future reference. You can then reuse this code in future projects, saving you time and effort.
How can I find the right jQuery Snippet for my needs?
There are many resources online where you can find jQuery snippets, including our own collection here at SitePoint. You can search these resources by keyword or browse by category to find snippets that match your needs. Always make sure to read the description and comments for each snippet to understand what it does and how to use it.
Are jQuery Snippets compatible with all browsers?
While jQuery itself is designed to be cross-browser compatible, individual jQuery snippets may not work perfectly in all browsers due to differences in how browsers interpret JavaScript. It’s always a good idea to test your code in multiple browsers to ensure it works as expected.
Can jQuery Snippets be used with other JavaScript libraries?
Yes, jQuery snippets can be used alongside other JavaScript libraries. However, you should be aware that using multiple libraries can sometimes lead to conflicts, as different libraries may use the same function or variable names. To avoid this, you can use jQuery’s noConflict() method, which allows you to use jQuery alongside other libraries without conflicts.
How can I troubleshoot a jQuery Snippet that’s not working?
If a jQuery snippet isn’t working, there are several steps you can take to troubleshoot the problem. First, check the console in your browser’s developer tools for any error messages. These messages can often provide clues about what’s going wrong. Next, make sure you’ve included the jQuery library in your project and that it’s being loaded before your snippet. Finally, check the syntax of your code to make sure there are no mistakes.
Can jQuery Snippets improve the performance of my website?
Yes, using jQuery snippets can potentially improve the performance of your website. By using pre-written, optimized code, you can accomplish tasks more efficiently and reduce the amount of code needed in your project. However, it’s important to remember that performance also depends on many other factors, such as the overall structure of your code and the speed of your server.
Where can I learn more about jQuery and jQuery Snippets?
There are many resources available online for learning about jQuery and jQuery snippets. The official jQuery website is a great place to start, as it provides comprehensive documentation and tutorials. Other resources include online coding platforms, blogs, and forums where you can learn from other developers and share your own knowledge.
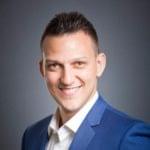
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.