- Key Takeaways
- Understanding Node CSV and Its Importance
- Getting Started with Node CSV
- Advanced Parsing Techniques with Node CSV
- Writing and Transforming CSV Data
- Integrating Node CSV with Node.js Streams
- Performance Considerations for Large CSV Datasets
- Real-World Applications of Node CSV
- Troubleshooting Common Node CSV Issues
- Extending Node CSV with Plugins and Community Contributions
- Summary
- Frequently Asked Questions
Efficiently manage CSV files in Node.js using Node CSV. This article cuts to the chase, offering you actionable solutions—from quick installation to effective file operations—tailored for developers seeking to optimize their CSV workflows in Node.js.
Key Takeaways
- Node CSV is a versatile parsing tool for handling CSV data in Node.js, providing features like generation, transformation, and serialization for managing large datasets efficiently.
- It is straightforward to use Node CSV, beginning with installation using npm, and offers simple syntax for parsing and writing CSV data, also enabling stream transformations and handling custom delimiters.
- Node CSV performance is optimized for large datasets using Node.js streams, proving beneficial in real-world applications like big data and e-commerce, and can be extended through plugins and community contributions.
Understanding Node CSV and Its Importance
We’ll kick off by exploring the fundamentals of Node CSV, its features, and its significance in data management.
Node CSV essentially serves as a comprehensive set of tools that facilitates:
- the generation
- parsing
- transformation
- serialization
Working with comma separated values is essential when dealing with CSV data, especially when managing a csv dataset and handling a csv stream. One way to achieve this is by using a parser converting csv text from a csv text input through a pipeline csv process.
Imagine having a swiss army knife for managing all your CSV-related tasks; that’s what Node CSV is!
What is Node CSV?
Node CSV is an advanced CSV parser designed for the Node.js environment. It’s armed with a user-friendly API, making it a breeze to work with CSV files. It is a scalable framework that supports various module systems, including ECMAScript modules and CommonJS.
The ability of Node CSV to handle large datasets coupled with its intuitive API makes it a preferred choice for developers working with CSV files in Node.js.
The Significance of CSV Files in Data Management
CSV files play a crucial role in data management, thanks to their lightweight structure that simplifies comprehension and processing. They serve as the backbone for storing, manipulating, and exchanging tabular data across a myriad of applications and industries. For instance, they can be used to efficiently import and export crucial data, such as customer or order information, to and from databases.
This process aids organizations in moving and consolidating large volumes of data into targeted databases.
Advantages of Using Node CSV
Node CSV brings several advantages to the table, including the ability to read CSV text, parse large CSV files, and convert CSV data into other formats. It’s not just about ease of use, but also about efficiency and integration.
Node CSV can easily be integrated with other Node.js libraries and frameworks, making it a versatile tool in the Node.js ecosystem.
Getting Started with Node CSV
Having understood the nature and significance of Node CSV, let’s now explore its installation and basic usage. The process is straightforward, and with the right guidance, you’ll be parsing and managing CSV files in Node.js in no time.
Installation Process
The initial step in utilizing Node CSV involves installing the library in your Node.js project. By executing the npm install csv
command in your command-line interface, you’ll be installing all the necessary modules for parsing and writing CSV data. To verify the successful installation, try importing the module in your Node.js code and look out for any error messages. If you see none, you’ve successfully installed Node CSV!
Basic Usage and Syntax
Once Node CSV is installed, you’re ready to harness its capabilities. The basic syntax involves using Node.js streams to read a CSV file. You’ll need to import the necessary modules and then process the data as per your requirements. To read a CSV file, use the csv-parse module in conjunction with Node.js’s fs module to stream the data. To write, the csv-stringify module can be employed to convert data into CSV format.
Advanced Parsing Techniques with Node CSV
Node CSV extends beyond basic CSV parsing, offering a plethora of advanced capabilities. The toolset is equipped with advanced parsing techniques that can significantly enhance your data manipulation capabilities. Whether it’s dealing with custom delimiters or handling errors and exceptions, Node CSV has got you covered.
Custom Delimiters and Escape Characters
Parsing intricate CSV files that extend beyond typical commas is no longer a problem with Node CSV. The library provides the capability to utilize custom delimiters through the ‘delimiter’ option, which can be defined as any character string or a Buffer. Furthermore, the ‘escape’ option facilitates the specification of an escape character for quoted fields.
Handling Errors and Exceptions
Like any coding process, Node CSV also encompasses handling of errors. The library offers an exception handling approach that involves utilizing a try-catch block to capture errors during parsing. Additionally, Node CSV provides an CsvError class, derived from JavaScript’s Error class, to facilitate the identification of error types and effective error management.
Writing and Transforming CSV Data
Apart from parsing CSV data, Node CSV also facilitates writing and transforming CSV data. Whether you’re looking to generate CSV strings from arrays or objects, or need to perform stream transformations, Node CSV has features and techniques to help you accomplish these tasks with ease.
Generating CSV Strings from Arrays or Objects
Creating CSV strings from arrays or objects is a breeze with Node CSV. The library allows you to read data from a CSV file and transform it into arrays. Then, using a module from NPM, such as ‘node-csv’, you can create the CSV string from the arrays.
Stream Transformations
The concept of stream transformations involves the manipulation and modification of CSV data using the Node.js stream. This process includes parsing the CSV data into a stream of objects and subsequently applying transformations to those objects.
Integrating Node CSV with Node.js Streams
Node CSV and Node.js streams are a natural, efficient combination. The integration of Node CSV with Node.js streams allows for efficient and effective reading and writing of CSV files, making it a powerful combination for any developer working with CSV files.
Reading CSV Files with Readable Streams
Readable streams in Node.js are an abstract interface designed for the manipulation of streaming data, enabling the retrieval of data from various sources like files or network sockets. Node.js interacts with CSV files using readable streams by utilizing the fs module’s createReadStream() method to read the data from the CSV file and create a readable stream.
Subsequently, the stream can be piped to a CSV parser module, such as csv-parser or fast-csv, to parse the CSV data and execute operations on it.
Writing CSV Data with Writable Streams
Writable streams in Node.js serve as an abstraction for a destination where data can be written. CSV data can be written using writable streams in Node.js by utilizing libraries such as ‘csv-write-stream’. These libraries offer a CSV encoder stream that can write arrays of strings or JavaScript objects, generating appropriately escaped CSV data.
Performance Considerations for Large CSV Datasets
Managing large CSV datasets can pose challenges in terms of performance and memory management. However, Node CSV proves its worth in these scenarios, offering techniques to optimize performance and manage memory usage efficiently.
Efficient Memory Usage
The Node.js stream API provides a more efficient way of managing memory by processing data in chunks through streaming, instead of loading the entire CSV file into memory at once. This method effectively decreases memory usage and improves performance, particularly for large CSV files. The js streaming api simplicity makes it an attractive solution for developers.
Speed Optimization Techniques
Speed matters, especially when you’re dealing with large CSV datasets. Modules such as csv-parser or fast-csv have been specifically developed to facilitate efficient CSV parsing by employing stream-based processing to handle the file in segments. The Node.js stream API also enables the efficient processing of large CSV files in smaller chunks, leading to improved performance and reduced memory usage.
Real-World Applications of Node CSV
Node CSV isn’t confined to theory; it’s practically applied across various sectors, including big data consulting firms and e-commerce platforms. Its versatility and robust functionality make it a preferred choice for managing CSV data across different sectors.
Big Data Consulting Firm Use Cases
Big data consulting firms incorporate Node CSV into their workflow to handle a range of tasks such as:
- CSV generation
- Parsing
- Data transformation
- Serialization
It provides the capability for efficient import and export of inventory information, as well as the ability to update quantities and manage stock levels.
E-commerce Data Management
In the e-commerce sector, Node CSV is used for managing product information and employee details. It facilitates the efficient handling of financial data and patient information by enabling the seamless manipulation and integration of CSV-formatted data across different applications and systems.
Troubleshooting Common Node CSV Issues
As with any tool, you may face challenges when using Node CSV. But don’t worry! Whether it’s parsing errors or file reading and writing complications, this section will guide you through troubleshooting common Node CSV issues.
Resolving Parsing Errors
Parsing errors in Node CSV can be identified by examining the error code, which provides information about the type of error. Typical parsing errors may involve encountering unrecognized Unicode/illegal codepoints and other issues while utilizing the CSV parser. To address these errors, check the CSV file for formatting issues, validate the file with dedicated file validation functions, and ensure proper error handling during parsing.
Debugging File Reading/Writing Complications
File reading and writing issues are common when working with Node CSV. To address these issues, examine the CSV file in a text editor to detect formatting problems or unexpected characters. Also, using console.log statements to display file contents during Node.js execution can help verify that it is being read accurately.
Extending Node CSV with Plugins and Community Contributions
Node CSV extends beyond its core functionality. The open-source community has contributed various plugins and extensions that augment its functionality and add features not present in the core library.
Popular Packages and Extensions
Packages such as fast-csv, xlsx – SheetJS, papaparse, json-2-csv, and other extensions such as csvtojson, csv-parser, csv, and nest-csv-parser are commonly used to improve the functionality of Node CSV. By utilizing these packages and extensions, you can take your CSV data manipulation capabilities to the next level.
How to Contribute to Node CSV
If you’re looking to contribute to the Node CSV project, review the guidelines outlined in the CONTRIBUTING.md file located in the project’s GitHub repository. Once you’re familiar with the guidelines, you can contribute by writing code, submitting bug reports, and offering tutorials and guides on utilizing Node CSV.
Summary
In conclusion, Node CSV is a versatile and powerful tool for managing CSV data in Node.js. Whether you’re parsing large datasets, handling complex CSV files, or managing data for a big data consulting firm or an e-commerce platform, Node CSV has got you covered. With the right knowledge and practices, you can master Node CSV and turn CSV data management into a piece of cake.
Frequently Asked Questions
What is the advantage of using Node CSV over other CSV parsers?
The advantage of using Node CSV over other CSV parsers is its comprehensive suite of functionalities for managing CSV files, user-friendly API, and scalability, especially with large datasets. It offers features for generation, parsing, transformation, and serialization.
Can Node CSV handle large CSV files?
Yes, Node CSV can handle large CSV files by processing data in smaller chunks through streaming, leading to improved performance and reduced memory usage.
What are some practical applications of Node CSV in real-world scenarios?
Node CSV is utilized in big data consulting firms for importing and exporting data, as well as in e-commerce for managing product information and customer details. It serves practical applications in data management across various industries.
How can I contribute to the Node CSV project?
You can contribute to the Node CSV project by writing code, submitting bug reports, or offering tutorials and guides. Review the guidelines in the CONTRIBUTING.md file on the project’s GitHub repository.
What should I do if I encounter parsing errors while using Node CSV?
If you encounter parsing errors while using Node CSV, you should identify the error code, then check the CSV file for formatting issues, ensure proper error handling during parsing, and validate the file using dedicated file validation functions. This can help address the errors and improve the parsing process.
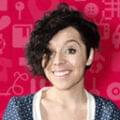
Dianne is SitePoint's newsletter editor. She especiallly loves learning about JavaScript, CSS and frontend technologies.