To get the a variables type using jQuery there is a jQuery function called .type() which returns “array”, “string”, “number”, “function”, “object” etc… At first thoughts I thought it must be very similar to the typeOf() JavaScript function. But as you can see in it’s implementation it’s using tostring() and class2type() checks. Interesting.
type: function( obj ) {
return obj == null ?
String( obj ) :
class2type[ toString.call(obj) ] || "object";
},
A closer look at the class2type object implementation.
var class2type = {
"[object Array]": "array",
"[object Boolean]": "boolean",
"[object Date]": "date",
"[object Function]": "function",
"[object Number]": "number",
"[object Object]": "object",
"[object RegExp]": "regexp",
"[object String]": "string"
};
Example use of jQuery.type() function.
var $forms = Array($('#register-form1'), $('#register-form2'), $('#register-form3'));
console.log($.type($forms));
//output: array
Frequently Asked Questions (FAQs) about jQuery Variable Type
What is the difference between jQuery.type() and JavaScript typeof?
jQuery.type() and JavaScript typeof are both used to determine the type of a variable, but they work in slightly different ways. JavaScript typeof is a unary operator that returns a string indicating the type of the unevaluated operand. However, it has limitations as it returns ‘object’ for null, arrays, and objects. On the other hand, jQuery.type() is a method that returns a string indicating the type of the evaluated operand. It can accurately identify the type of objects, arrays, null, and other data types.
How can I use jQuery.type() to check for a specific data type?
To use jQuery.type() to check for a specific data type, you can compare the returned string with the data type you are interested in. For example, if you want to check if a variable is an array, you can use the following code:if (jQuery.type(variable) === "array") {
// variable is an array
}
Can jQuery.type() detect custom object types?
Yes, jQuery.type() can detect custom object types. If the object is created using a constructor function, jQuery.type() will return the name of the constructor function. For example, if you have a custom object created with a constructor function named ‘MyObject’, jQuery.type() will return ‘MyObject’ when called with this object.
What is the return value of jQuery.type() for null and undefined?
jQuery.type() returns ‘null’ for null values and ‘undefined’ for undefined values. This is different from JavaScript typeof, which returns ‘object’ for null and ‘undefined’ for undefined.
Can jQuery.type() be used with jQuery objects?
Yes, jQuery.type() can be used with jQuery objects. When used with a jQuery object, it will return ‘object’. To get more specific information about the jQuery object, you can use methods provided by jQuery, such as .is() and .has().
Is jQuery.type() case-sensitive?
Yes, jQuery.type() is case-sensitive. It will return the type of the variable in lowercase. For example, it will return ‘number’ for a Number, ‘string’ for a String, and ‘object’ for an Object.
How does jQuery.type() handle NaN?
jQuery.type() treats NaN (Not a Number) as a number. This is because in JavaScript, NaN is considered a special number value.
Can jQuery.type() detect the difference between an array and an object?
Yes, jQuery.type() can accurately differentiate between an array and an object. While JavaScript typeof returns ‘object’ for both arrays and objects, jQuery.type() returns ‘array’ for arrays and ‘object’ for objects.
Is jQuery.type() available in all versions of jQuery?
jQuery.type() was added in jQuery 1.4.3. It is available in all subsequent versions of jQuery.
Can jQuery.type() be used to check the type of a function?
Yes, jQuery.type() can be used to check the type of a function. When used with a function, it will return ‘function’.
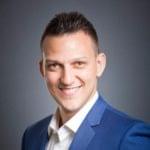
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.