If you’ve been following this series you’ll know what SVGs are, why you should consider them, how to create basic drawings and six ways to embed SVGs in your web page. While creating SVG XML may be easy, the code can be a little verbose, e.g.
<circle cx="100" cy="300" r="80"
stroke="#909" stroke-width="10" fill="#f6f" />
Wouldn’t it be great if we could apply CSS styling rules to SVG elements? Well, that’s exactly what you can do! Instead of attributes, you can use inline styles with identical property names:
<circle cx="100" cy="100" r="80"
style="stroke: #909; stroke-width: 10; fill: #f6f;" />
Inline styles are still clunky so you can embed stylesheet code. Standard CSS selectors are used to apply styles to element types or those with specific IDs or class names, e.g.
<?xml version="1.0" encoding="UTF-8" standalone="no"?>
<!DOCTYPE svg PUBLIC "-//W3C//DTD SVG 1.1//EN" "https://www.w3.org/Graphics/SVG/1.1/DTD/svg11.dtd">
<svg xmlns="https://www.w3.org/2000/svg" viewBox="0 0 200 200" preserveAspectRatio="xMidYMid meet">
<defs>
<style type="text/css"><![CDATA[
circle {
stroke: #909;
stroke-width: 10;
fill: #f6f;
}
]]></style>
</defs>
<circle cx="100" cy="100" r="80" />
</svg>
Note that the <![CDATA[ … ]]> block is required since CSS can contain > characters which will confuse XML parsers. I recommend you use them regardless.
That’s great but, as all good developers know, it’s best to separate your stylesheets so they’re easier to maintain and can be reused elsewhere. We can do that by adding a new xml-stylesheet tag immediately after the XML declaration:
<?xml version="1.0" encoding="UTF-8" standalone="no"?>
<?xml-stylesheet href="styles.css" type="text/css"?>
<!DOCTYPE svg PUBLIC "-//W3C//DTD SVG 1.1//EN" "https://www.w3.org/Graphics/SVG/1.1/DTD/svg11.dtd">
<svg...
Creating the SitePoint Logo as a CSS-Styled SVG
SVGs are ideal for logos since they can be scaled to any size and used in a responsive template. We’ll start by defining a sitepoint.svg file which points to a stylesheet named sp-styles.css:
<?xml version="1.0" encoding="UTF-8" standalone="no"?>
<?xml-stylesheet href="sp-styles.css" type="text/css"?>
<!DOCTYPE svg PUBLIC "-//W3C//DTD SVG 1.1//EN" "https://www.w3.org/Graphics/SVG/1.1/DTD/svg11.dtd">
<svg xmlns="https://www.w3.org/2000/svg" viewBox="95 40 550 180" preserveAspectRatio="xMidYMid meet">
<title>SitePoint</title>
<desc>The SitePoint Logo</desc>
<g id="main">
We’ll follow this with polyline elements for the blue slants but won’t worry about styling just yet:
<polyline points="100,100 150,100 170,150 120,150" />
<polyline points="170,100 220,100 240,150 190,150" />
The orange slants are defined using similar polylines except that we’ll give them a class named “orange”:
<polyline class="orange" points="120,45 170,45 150,95 100,95" />
<polyline class="orange" points="190,155 240,155 220,205 170,205" />
We finish with the logo text and closing SVG tags:
<text id="sp" x="240" y="150">sitepoint</text>
</g>
</svg>
The unstyled SVG can be viewed in your browser:
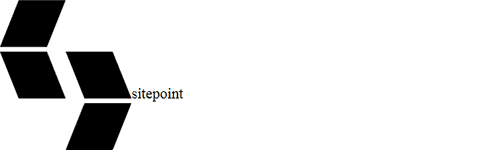
polyline
{
stroke: 0;
stroke-linejoin: butt;
fill: #003565;
}
Polylines with a class name of “orange” will have an orange fill applied:
polyline.orange
{
fill: #ff6400;
}
Finally, we can apply simple text styling. We could use webfonts or any of the standard CSS font/text properties:
text#sp
{
font-family: verdana, sans-serif;
font-size: 570%;
fill: #003565;
}
Our SVG is now complete:
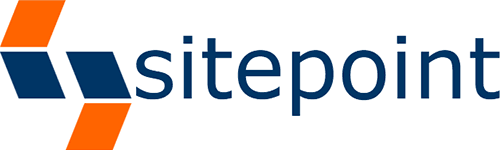
Using the SVG in a Responsive Design
A simple HTML5 template can be developed to display the SVG in anobject
tag:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<title>SVG logo</title>
</head>
<style>
html, body
{
width: 100%;
height: 100%;
overflow: hidden;
}
#sp
{
display: block;
width: 100%;
height: 100%;
margin: auto;
}
</style>
<body>
<object id="sp" type="image/svg+xml" data="sitepoint.svg">SitePoint</object>
</body>
</html>
It doesn’t matter how the browser height or width dimensions change, the logo will always appear in the center of the page at the maximum possible size. The quality remains good regardless.
View the SVG logo demonstration page…
The combined size of the SVG and CSS file is 931 bytes before compression and gzipping. The PNG shown above won’t look good above a 500 pixel width and has a file size of 3.4kB after compression
… (although it will work in IE6/7/8!).
Stay tuned to SitePoint for more SVG articles coming soon…
And if you enjoyed reading this post, you’ll love Learnable; the place to learn fresh skills and techniques from the masters. Members get instant access to all of SitePoint’s ebooks and interactive online courses, like HTML5 & CSS3 For the Real World.
Comments on this article are closed. Have a question about CSS? Why not ask it on our forums?
Frequently Asked Questions on SVG Styling with CSS
How can I use CSS to change the color of an SVG image?
Changing the color of an SVG image using CSS is quite straightforward. You can use the ‘fill’ property to change the color of the SVG. Here’s an example:svg {
fill: #ff0000; /* This will change the SVG color to red */
}
Remember, the ‘fill’ property only changes the color of the SVG shapes, not the strokes or lines.
Can I use CSS to animate SVGs?
Yes, you can animate SVGs using CSS. SVGs are made up of elements that can be manipulated using CSS animations and transitions. For instance, you can animate the position, size, rotation, and color of SVG elements. Here’s a simple example of a CSS animation applied to an SVG:@keyframes spin {
from { transform: rotate(0deg); }
to { transform: rotate(360deg); }
}
svg {
animation: spin 2s linear infinite;
}
This will make the SVG spin indefinitely.
How can I style SVGs with external CSS?
To style SVGs with external CSS, you need to include the SVG within the HTML document. External CSS cannot style SVGs that are included as an image source. Once the SVG is included in the HTML, you can target it with CSS selectors just like any other HTML element.
Can I use media queries with SVGs?
Yes, you can use media queries with SVGs. This allows you to change the style of the SVG based on the viewport size. This can be useful for creating responsive designs where the SVG changes size, color, or even shape based on the screen size.
How can I use CSS to change the stroke of an SVG?
You can use the ‘stroke’ and ‘stroke-width’ properties to change the stroke color and width of an SVG. Here’s an example:svg {
stroke: #000000; /* This will change the stroke color to black */
stroke-width: 2; /* This will change the stroke width to 2 pixels */
}
Can I use CSS to control the opacity of an SVG?
Yes, you can control the opacity of an SVG using the ‘opacity’ property in CSS. This can be useful for creating effects where the SVG fades in or out.
How can I use CSS to scale an SVG?
You can use the ‘transform’ property in CSS to scale an SVG. Here’s an example:svg {
transform: scale(2); /* This will double the size of the SVG */
}
Can I use CSS to position an SVG?
Yes, you can use CSS to position an SVG. You can use the ‘position’ property along with ‘top’, ‘right’, ‘bottom’, and ‘left’ properties to position the SVG.
How can I use CSS to rotate an SVG?
You can use the ‘transform’ property in CSS to rotate an SVG. Here’s an example:svg {
transform: rotate(45deg); /* This will rotate the SVG 45 degrees */
}
Can I use CSS to skew an SVG?
Yes, you can use the ‘transform’ property in CSS to skew an SVG. Here’s an example:svg {
transform: skewX(20deg); /* This will skew the SVG 20 degrees along the X-axis */
}
Craig is a freelance UK web consultant who built his first page for IE2.0 in 1995. Since that time he's been advocating standards, accessibility, and best-practice HTML5 techniques. He's created enterprise specifications, websites and online applications for companies and organisations including the UK Parliament, the European Parliament, the Department of Energy & Climate Change, Microsoft, and more. He's written more than 1,000 articles for SitePoint and you can find him @craigbuckler.