Quick Tip: Creating a Date Picker in React
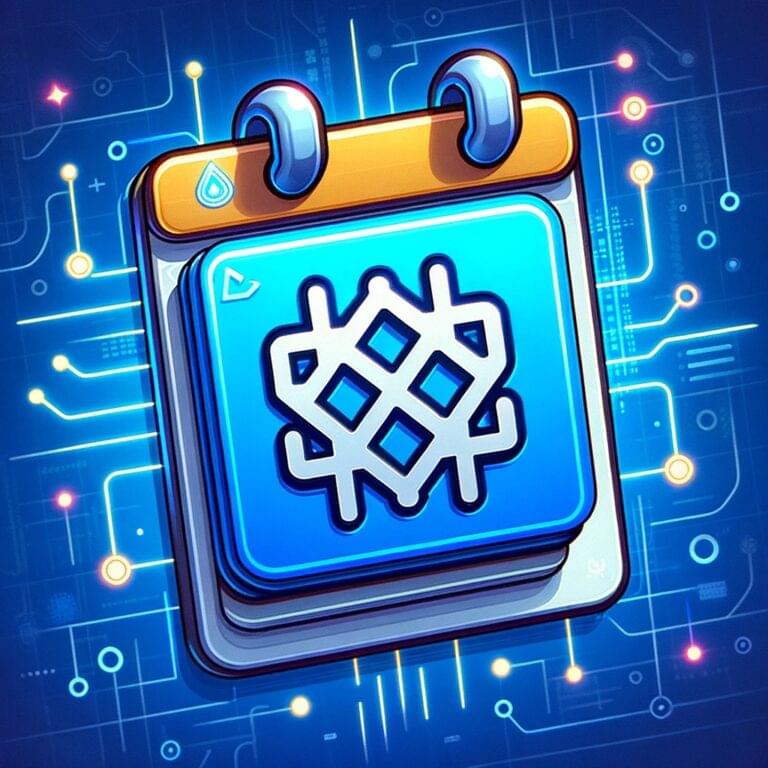
When developing a React application, you may need a way for users to select dates — whether for a booking system, a form, or a calendar. One of the most user-friendly ways to do this is by implementing a date picker. This guide will walk you through the process of adding a date picker to your React application using the react-datepicker
library.
Step 1: Set Up Your React Project
Before starting, make sure you have a React project set up. If you don’t have one, you can create one quickly by running npx create-react-app my-datepicker-app
. (Read about Create React App to learn more.)
Step 2: Install react-datepicker
The first step is to add the react-datepicker
package to your project. Open your terminal, navigate to your project directory, and run the following command:
npm install react-datepicker
This command installs react-datepicker
and its dependencies into your project.
Step 3: Import react-datepicker
With the package installed, you can now use it in your React component. Open the component file where you want to include the date picker, and add the following import statement at the top:
import DatePicker from "react-datepicker";
import "react-datepicker/dist/react-datepicker.css";
The first line imports the DatePicker
component, and the second line imports the default styling. This is crucial for the date picker to render correctly.
Step 4: Using DatePicker
in Your Component
Now, let’s add the DatePicker
component to your render method or return statement if you’re using a functional component. You’ll also need to manage the selected date in your component’s state.
Here’s how you can do it in a class component:
import React, { Component } from 'react';
import DatePicker from "react-datepicker";
import "react-datepicker/dist/react-datepicker.css";
class MyDatePicker extends Component {
state = {
// Initially, no date is selected
startDate: null
};
handleChange = date => {
this.setState({
startDate: date
});
};
render() {
return (
<DatePicker
selected={this.state.startDate}
onChange={this.handleChange}
/>
);
}
}
export default MyDatePicker;
For a functional component using hooks, the code looks like this:
import React, { useState } from 'react';
import DatePicker from "react-datepicker";
import "react-datepicker/dist/react-datepicker.css";
const MyDatePicker = () => {
const [startDate, setStartDate] = useState(null);
return (
<DatePicker
selected={startDate}
onChange={(date) => setStartDate(date)}
/>
);
};
export default MyDatePicker;
Step 5: Customization and Options
react-datepicker
offers a wide range of props to customize the date picker’s appearance and functionality. Some of the customization options include:
- dateFormat: Allows you to change the format of the displayed date.
- minDate and maxDate: Restrict the selectable date range.
- inline: Render the date picker inline instead of as a dropdown.
- withPortal: Renders the date picker inside a portal, which can help with positioning issues in complex layouts.
Here’s an example of a customized DatePicker
:
<DatePicker
selected={startDate}
onChange={date => setStartDate(date)}
dateFormat="yyyy/MM/dd"
minDate={new Date()}
maxDate={new Date().setMonth(new Date().getMonth() + 1)}
inline
/>
Issues to Watch Out For
When creating a date picker in React applications, focusing on several key issues can enhance usability, accessibility, and functionality:
- Browser compatibility. Ensure consistent operation across all target browsers, as differences can affect the date picker’s behavior and appearance.
- Responsive design. The date picker should work seamlessly across devices of various screen sizes, ensuring a good user experience on both desktops and mobile devices.
- Accessibility. Implement keyboard navigation, screen reader support, and ARIA attributes to make the date picker accessible to all users, including those with disabilities.
- Localization and internationalization. Support different languages and date formats to cater to a global audience, ensuring the date picker meets the cultural and linguistic expectations of your users.
- Time zone handling. Accurately handle time zones, especially for applications used across different regions, to avoid confusion and errors in date selection.
- Performance. Be mindful of the impact on your application’s performance, optimizing as necessary to maintain a smooth and responsive experience.
- Dependency management. Regularly update the date picker library to benefit from security patches and new features, but test thoroughly to avoid introducing new issues.
Conclusion
Integrating a date picker in your React application can significantly improve the user experience by providing a simple and effective way to select dates. The react-datepicker
library makes it straightforward to add a customizable and stylish date picker to your app with just a few lines of code.
Experiment with react-datepicker
‘s various props and options to fully utilize its potential and tailor the date picker to fit your application’s needs perfectly.
Dianne is SitePoint's newsletter editor. She especiallly loves learning about JavaScript, CSS and frontend technologies.