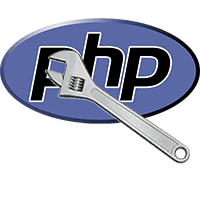
Loading an Extension
For the purposes of this article, we’ll assume you need to create or manipulate images and require PHP’s GD library. To load the GD library on your system:- For Windows, you need to include/uncomment the line extension=php_gd2.dll in your php.ini configuration file.
- For Linux/Unix, you should use the PHP configure option
--with-gd
. - For Mac OS X … er, you best Google it. Apologies for not providing specific details, but there appear to be multiple ways of configuring GD support. It depends on your version of OS X and whether you’re using the built-in web server or a custom installation of Apache.
Checking an Extension is Loaded
PHP provides an extension_loaded(name) function which returns true when the named library is available, e.g.
<?php
if (extension_loaded('gd')) {
echo 'GD extension is loaded and everything is fine!';
}
else {
echo 'Where is the GD library?';
exit();
}
?>
Alternatively, you can check for the existence of specific library function using function_exists(), e.g.
<?php
if (function_exists('gd_info')) {
echo 'gd_info() is available so the GD library is probably available.';
}
else {
echo 'gd_info() cannot be found?';
exit();
}
?>
However, function_exists() is more risky — another developer could write their own function named ‘gd_info’.
I’d recommend using function_exists() in situations when a function has been introduced in a later version of PHP. For example, if your application runs in both PHP4 and PHP5, you could check for the existance of imagefilter() (a PHP5-only GD function) before attempting to modify an image.
Handling Unloaded Extensions
PHP provides a dl() function for dynamically loading extensions at runtime. Unfortunately:- it may be disabled on your system
- extensions must be loaded by referencing the filename; these are different across platforms
- it’s a little flaky…
- so it’s has been deprecated in PHP 5.3
- and will be removed from PHP6.
- Ensure the extension is enabled on all your target platforms before coding begins!
- Alert the administrator when an essential extension is not available during installation. For example, your application should stop gracefully and provide further instructions if it won’t run without the PDO database interface.
- Provide a downgraded experience. For example, your application could disable image manipulation if the GD library is not available. You could alert the administrator during installation but still let the application run.
Frequently Asked Questions (FAQs) about PHP Extensions
What are the benefits of using PHP extensions?
PHP extensions are a powerful tool that can significantly enhance the functionality of your PHP applications. They provide a way to add new features and capabilities to PHP without having to modify the core PHP code. This can include everything from adding support for new databases, improving performance through caching, or even adding new language features. By using PHP extensions, developers can customize their PHP environment to better suit their specific needs and requirements.
How can I install a PHP extension?
Installing a PHP extension typically involves downloading the extension’s source code, compiling it, and then adding it to your PHP configuration. The exact steps can vary depending on the specific extension and your server environment. However, many popular PHP extensions are included with the standard PHP distribution and can be easily enabled through the PHP configuration file.
Why is my PHP extension not loading?
There could be several reasons why a PHP extension is not loading. The most common reason is that the extension is not properly installed or configured. This could be due to an error during the installation process, or it could be that the extension is not enabled in your PHP configuration file. Other potential issues could include compatibility problems with your PHP version or conflicts with other extensions.
How can I check if a PHP extension is loaded?
You can check if a PHP extension is loaded by using the extension_loaded()
function in PHP. This function takes the name of the extension as a parameter and returns a boolean value indicating whether the extension is loaded or not. For example, extension_loaded('mysqli')
would check if the MySQLi extension is loaded.
Can I create my own PHP extension?
Yes, it is possible to create your own PHP extensions. This requires a good understanding of both PHP and C, as PHP extensions are typically written in C. The PHP website provides a detailed guide on how to create your own extensions, including how to structure your code, how to compile your extension, and how to add it to your PHP configuration.
What are some common PHP extensions and what do they do?
There are many PHP extensions available, each providing different functionality. Some common ones include MySQLi, which provides a way to interact with MySQL databases; GD, which is used for creating and manipulating image files; and mbstring, which provides functions for working with multibyte strings.
How can I enable or disable a PHP extension?
PHP extensions can be enabled or disabled through the PHP configuration file, php.ini
. To enable an extension, you need to add or uncomment a line in the configuration file that refers to the extension. To disable an extension, you can comment out the line referring to the extension or remove it entirely.
Are there any risks associated with using PHP extensions?
While PHP extensions can provide many benefits, they can also introduce potential risks. For example, poorly written or outdated extensions can introduce security vulnerabilities or cause stability issues. Therefore, it’s important to only use extensions from trusted sources and to keep them up to date.
Can PHP extensions improve the performance of my application?
Yes, some PHP extensions are specifically designed to improve performance. For example, the OPcache extension can significantly speed up PHP by caching precompiled script bytecode, while the APCu extension provides a way to cache data in memory, reducing the need for expensive database queries.
What is the difference between built-in and PECL extensions?
Built-in extensions are included with the standard PHP distribution and are maintained by the PHP team. PECL extensions, on the other hand, are maintained by the PHP community and are not included with the standard PHP distribution. They can be downloaded and installed separately. Both types of extensions can provide valuable functionality, but PECL extensions may not be as thoroughly tested or as stable as built-in extensions.
Craig is a freelance UK web consultant who built his first page for IE2.0 in 1995. Since that time he's been advocating standards, accessibility, and best-practice HTML5 techniques. He's created enterprise specifications, websites and online applications for companies and organisations including the UK Parliament, the European Parliament, the Department of Energy & Climate Change, Microsoft, and more. He's written more than 1,000 articles for SitePoint and you can find him @craigbuckler.