Introduction
We are in an age where, typically, an application cannot work in total isolation and does not have all the data it needs to do its work. It generally has to communicate to some other application–maybe over the internet–and read or send some data to it. However, the applications with which your app communicates might not be developed using the same technology as yours. For smooth data exchanges between your app and some other application you might be exchanging data in an open format like Extensible Markup language or XML. An XML document is a human readable text document that contains some starting and ending tags with attributes and data in those tags. Most of the languages and platforms have support for parsing and creating XML documents in them. Android also provides us APIs so that we can work and process XML documents in our app with ease and flexibility. In this article, we are going to see how you can work effectively with XML documents in your Android app.The classes for XML parsing in Android
There are various XML parsers we can use on the Android platform. For XML, Android recommends the use of theXMLPullParser
. Android gives a multiple implementation of the XMLPullParser
and you can use any of them in your program. Depending on the way you create your XMLPullParser
, the implementation of the parser will be chosen.
Instantiating your parser
As stated above, there are multiple ways in Android you can create anXMLPullParser
. The first way to do it is using the Xml class as shown below:
xmlpullparser parser = Xml.newPullParser();You have to just call
newPullParser
which will return you a reference to the XMLPull
parser. Or you can get a reference from the XmlPullParserFactory
as shown below.
XmlPullParserFactory pullParserFactory; try { pullParserFactory = XmlPullParserFactory.newInstance(); XmlPullParser parser = pullParserFactory.newPullParser(); } catch (XmlPullParserException e) { e.printStackTrace(); }
Parsing the XML
Once we have the parser reference it’s now time to use it to parse an XML. We are going to use the following XML as the XML to parse. So now create a file calledtemp.xml
in your assets folder with the content:
<?xml version="1.0" encoding="UTF-8"?> <products> <product> <productname>Jeans</productname> <productcolor>red</productcolor> <productquantity>5</productquantity> </product> <product> <productname>Tshirt</productname> <productcolor>blue</productcolor> <productquantity>3</productquantity> </product> <product> <productname>shorts</productname> <productcolor>green</productcolor> <productquantity>4</productquantity> </product> </products>The code of the activity to read and parse is as follows:
class Product { public String name; public String quantity; public String color; } public class XMLDemo extends Activity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); XmlPullParserFactory pullParserFactory; try { pullParserFactory = XmlPullParserFactory.newInstance(); XmlPullParser parser = pullParserFactory.newPullParser(); InputStream in_s = getApplicationContext().getAssets().open("temp.xml"); parser.setFeature(XmlPullParser.FEATURE_PROCESS_NAMESPACES, false); parser.setInput(in_s, null); parseXML(parser); } catch (XmlPullParserException e) { e.printStackTrace(); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } } private void parseXML(XmlPullParser parser) throws XmlPullParserException,IOException { ArrayList<product> products = null; int eventType = parser.getEventType(); Product currentProduct = null; while (eventType != XmlPullParser.END_DOCUMENT){ String name = null; switch (eventType){ case XmlPullParser.START_DOCUMENT: products = new ArrayList(); break; case XmlPullParser.START_TAG: name = parser.getName(); if (name == "product"){ currentProduct = new Product(); } else if (currentProduct != null){ if (name == "productname"){ currentProduct.name = parser.nextText(); } else if (name == "productcolor"){ currentProduct.color = parser.nextText(); } else if (name == "productquantity"){ currentProduct.quantity= parser.nextText(); } } break; case XmlPullParser.END_TAG: name = parser.getName(); if (name.equalsIgnoreCase("product") && currentProduct != null){ products.add(currentProduct); } } eventType = parser.next(); } printProducts(products); } private void printProducts(ArrayList</product><product> products) { String content = ""; Iterator</product><product> it = products.iterator(); while(it.hasNext()) { Product currProduct = it.next(); content = content + "nnnProduct :" + currProduct.name + "n"; content = content + "Quantity :" + currProduct.quantity + "n"; content = content + "Color :" + currProduct.color + "n"; } TextView display = (TextView)findViewById(R.id.info); display.setText(content); } @Override public boolean onCreateOptionsMenu(Menu menu) { // Inflate the menu; this adds items to the action bar if it is present. getMenuInflater().inflate(R.menu.main, menu); return true; } }In the above code we have created a small class called Product which has three members: name, quantity and color. In the activity we first get the
XMLPullParser
instance then we open the temp.xml
as an inputstream
. Once that is done we set the inputstream
as input to the parser. Then we parse the xml in our function parseXML
.
In the function parseXML
we create an ArrayList
in which we will store all the products which we will parse from the XML. Once that is done we create a loop which will work till the end of the document is reached.
At the start of the document we created the ArrayList
of products. If a tag starts and if the tag is product we know that now it’s a new product so we create a new product instance. Then if the other tags are read we will just read and add the values in our Product instance.
Once the Product tag is ended we add the product to the ArrayList
. Once all the products are added we call the function printProducts
in which we iterate over the ArrayList
and then print the values on the screen.
If we run the activity now we will see the output as follows:
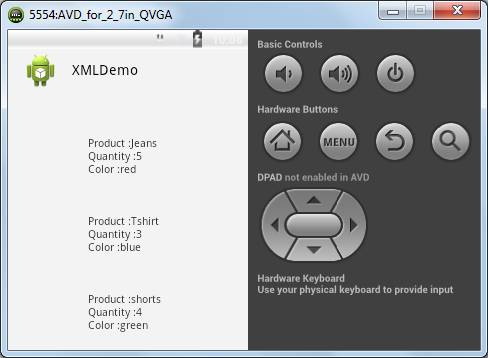
Other Parsers used for XML
DOM
There are other parsers also that can be used to parse XML in Android. The DOM parser can be used which creates a complete memory model of the XML and can be used to either create or parse XML. DOM parsers generally consume a lot of memory. You can get the instance of aDOMBuilder
as shown below
try { DocumentBuilderFactory DOMfactory = DocumentBuilderFactory.newInstance(); DocumentBuilder DOMbuilder = DOMfactory.newDocumentBuilder(); } catch (ParserConfigurationException e) { e.printStackTrace(); }
SAX Parsers
TheSAXParsers
can also be used to parse XML and consume much lesser memory then DOM parsers. The SAXParsers
can be used only to parse XMLs not to create them. You can get the reference of a SAXParser
as shown below:
SAXParserFactory SAXfactory = SAXParserFactory.newInstance(); try { SAXParser SAXParserObj = SAXfactory.newSAXParser(); } catch (Exception e) { e.printStackTrace(); }
Conclusion
XML documents are becoming a standard way to exchange data between applications over the internet. With our Android apps becoming smarter and smarter it might be necessary for your Android app to communicate and exchange data between different applications over the internet. Android provides a rich set of APIs to work with XML documents in your Android app. With the help of these APIs you can easily incorporate XML into your Android app. The XML parsers do the tedious work of parsing the XML and we have to just write the code to fetch the appropriate information from it and store it for further processing. So, have fun processing XML in your next Android app. And if you enjoyed reading this post, you’ll love Learnable; the place to learn fresh skills and techniques from the masters. Members get instant access to all of SitePoint’s ebooks and interactive online courses, like Beginning Android Development. Comments on this article are closed. Have a question about developing Apps? Why not ask it on our forums?Frequently Asked Questions (FAQs) about Parsing XML Data in Android Apps
What are the different types of XML parsers available in Android?
Android provides three types of XML parsers which are DOM, SAX, and XMLPullParser. DOM parser is a tree-based parser that loads the entire XML document into memory and then allows you to navigate and manipulate the tree structure. SAX parser is an event-based parser that reads XML data as a stream and triggers events when it encounters certain elements. XMLPullParser is a pull parsing method for XML parsing which is more efficient and easier to use than DOM and SAX parsers.
How does XMLPullParser work in Android?
XMLPullParser is an interface that defines parsing functionality. It works by iterating through the XML document and generating ‘events’ when it encounters certain elements. For example, when the parser encounters a start tag, an end tag, or text between tags, it generates corresponding events. The programmer can then define what actions to take upon these events, such as storing the data in variables.
What are the advantages of using DOM parser in Android?
DOM parser loads the entire XML document into memory and creates a tree structure. This allows for easy navigation and manipulation of the XML data. You can add, modify, and delete elements. DOM parser is also language independent and comes with a lot of flexibility. However, it can be memory intensive if the XML document is large.
How can I handle exceptions while parsing XML in Android?
Exceptions can be handled in XML parsing by using try-catch blocks. For instance, you can catch a SAXException or an IOException when using a SAX parser. These exceptions can occur if there is an error in the XML document or if there is an issue with the input/output operations.
How can I parse XML data from a URL in Android?
To parse XML data from a URL, you first need to establish a connection to the URL using HttpURLConnection or HttpClient. Then, you can use an InputStream to read the data from the URL. Once you have the data, you can use an XML parser to parse the data.
How can I use namespaces while parsing XML in Android?
Namespaces in XML are used to avoid element name conflicts. In Android, you can use namespaces while parsing XML by using the methods provided by the XML parser. For instance, XMLPullParser provides the getNamespace() method to get the current namespace.
How can I parse XML data stored in a string in Android?
To parse XML data stored in a string, you can use a StringReader to read the string and then use an XML parser to parse the data. For instance, you can use XMLPullParser with a StringReader to parse XML data stored in a string.
How can I parse XML data stored in a file in Android?
To parse XML data stored in a file, you can use a FileReader or FileInputStream to read the file and then use an XML parser to parse the data. For instance, you can use XMLPullParser with a FileReader to parse XML data stored in a file.
How can I parse XML data from a network response in Android?
To parse XML data from a network response, you can use an InputStream to read the response and then use an XML parser to parse the data. For instance, you can use XMLPullParser with an InputStream to parse XML data from a network response.
How can I parse XML data with attributes in Android?
XML elements can have attributes that provide additional information about the elements. In Android, you can parse XML data with attributes by using the methods provided by the XML parser. For instance, XMLPullParser provides the getAttributeValue() method to get the value of an attribute.
Abbas is a software engineer by profession and a passionate coder who lives every moment to the fullest. He loves open source projects and WordPress. When not chilling around with friends he's occupied with one of the following open source projects he's built: Choomantar, The Browser Counter WordPress plugin, and Google Buzz From Admin.