- Why Use For Loops in JavaScript Code
- Definition of the for…in Loop
- Syntax of the for…in Loop
- Using the for Loop with Objects
- Using a for…in Loop with Arrays
- Using a for…in Loop with Strings
- When Not to Use a JavaScript for…in Loop
- Alternatives to For Loops in JavaScript
- Conclusion
- FAQs about the for loop in JavaScript
Loops allow us to cycle through items in arrays or objects and do things like print them, modify them, or perform other kinds of tasks or actions. There are different kinds of loops, and the for loop in JavaScript allows us to iterate through a collection (such as an array).
In this article, we’ll learn about the for
loop JavaScript provides. We’ll look at how for...in
loop statements are used in JavaScript, the syntax, examples of how it works, when to use or avoid it, and what other types of loops we can use instead.
Why Use For Loops in JavaScript Code
In JavaScript, just as in other programming languages, we use loops to read or access the items of a collection. The collection can be an array or an object. Every time the loop statement cycles through the items in a collection, we call it an iteration.
There are two ways to access an item in a collection. The first way is via its key in the collection, which is an index in an array or a property in an object. The second way is via the item itself, without needing the key.
Definition of the for…in Loop
The JavaScript for
loop goes through or iterates keys of a collection. Using these keys, you can then access the item it represents in the collection.
The collection of items can be either arrays, objects, or even strings.
Syntax of the for…in Loop
The for
loop has the following syntax or structure:
for (let key in value) {
//do something here
}
In this code block, value
is the collection of items we’re iterating over. It can be an object, array, string, and so on. key
will be the key of each item in value
, changing on each iteration to the next key in the list.
Note that we use let
or const
to declare key
.
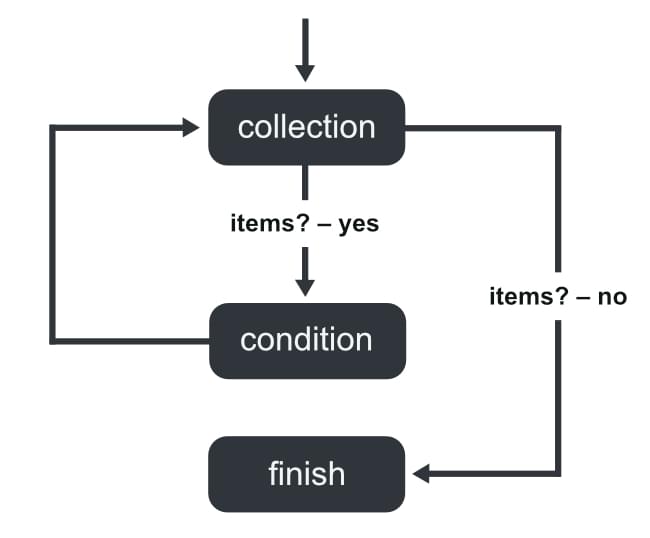
Using the for Loop with Objects
When using for...in
loop to iterate an object in JavaScript, the iterated keys or properties — which, in the snippet above, are represented by the key
variable — are the object’s own properties.
As objects might inherit items through the prototype chain, which includes the default methods and properties of Objects as well as Object prototypes we might define, we should then use hasOwnProperty.
For loop example over an iterable object
In the following example, we’re looping over the variable obj
and logging each property and value:
const obj = {
"a": "JavaScript",
1: "PHP",
"b": "Python",
2: "Java"
};
for (let key in obj) {
console.log(key + ": " + obj[key] )
}
// Output:
// "1: PHP"
// "2: Java"
// "a: JavaScript"
// "b: Python"
Notice that the order of the iteration is ascending for the keys (that is, starting with digits in numerical order and then letters in alphabetical order). However, this outputted order is different from the index order of the items as created when initializing the object.
See the Pen Looping Objects by SitePoint (@SitePoint) on CodePen.
Using a for…in Loop with Arrays
When using the for...in
loop to iterate arrays in JavaScript, key
in this case will be the indices of the elements. However, the indices might be iterated in a random order.
So, if the value
variable in the for...in
loop syntax structure we showed above was an array of five items, key
would not be guaranteed to be 0 to 4. Some indices might precede others. Details on when this might happen is explained later in this article.
For…in loop array example
In the example below, we’re looping over the following variable arr
:
const arr = ["JavaScript", "PHP", "Python", "Java"];
for (let key in arr) {
console.log(key + ": " + arr[key])
}
// Output:
// "0: JavaScript"
// "1: PHP"
// "2: Python"
// "3: Java"
And in the loop, we’re rendering the index and the value of each array element.
See the Pen Looping Arrays by SitePoint (@SitePoint) on CodePen.
Using a for…in Loop with Strings
You can loop over a string with the JavaScript for...in
loop. However, it’s not recommended to do so, as you’ll be looping over the indices of the characters rather than the characters themselves.
A for…in loop string example
In the example below, we’re looping over the following variable str
:
const str = "Hello!";
for (let key in str) {
console.log(key + ": " + str.charAt(key));
}
//Output
// "0: H"
// "1: e"
// "2: l"
// "3: l"
// "4: o"
// "5: !"
Inside the loop, we’re rendering the key, or index of each character, and the character at that index.
See the Pen Strings Loop by SitePoint (@SitePoint) on CodePen.
Let’s look at the situations that the JavaScript for...in
loop is best suited to.
Iterating objects with a JavaScript for…in loop
Because the for...in
loop only iterates the enumerable properties of an object — which are the object’s own properties rather than properties like toString
that are part of the object’s prototype — it’s good to use a for...in
loop to iterate objects. A for...in
loop provides an easy way to iterate over an object’s properties and ultimately its values.
Debugging with a for…in loop
Another good use case for the JavaScript for...in
loop is debugging. For example, you may want to print to the console or an HTML element the properties of an object and its values. In this case, the for...in
loop is a good choice.
When using the for...in
loop for debugging objects and their values, you should always keep in mind that iterations are not orderly, meaning that the order of items that the loop iterates can be random. So, the order of the properties accessed might not be as expected.
When Not to Use a JavaScript for…in Loop
Now let’s look at situations where a for...in
loop isn’t the best option.
Orderly iterating of arrays
As the index order in the iterations is not guaranteed when using the for...in
loop, it’s recommended to not iterate arrays if maintaining the order is necessary.
This is especially essential if you’re looking to support browsers like IE, which iterates in the order the items are created rather than in the order of the indices. This is different from the way current modern browsers work, which iterate arrays based on the indices in ascending order.
So, for example, if you have an array of four items and you insert an item into position three, in modern browsers the for...in
loop will still iterate the array in order from 0 to 4. In IE, when using a for...in
loop, it will iterate the four items that were originally in the array in the beginning and then reach the item that was added at position three.
Making changes while iterating
Any addition, deletion, or modification to properties doesn’t guarantee an ordered iteration. Making changes to properties in a for...in
loop should be avoided. This is mostly due to its unordered nature.
So, if you delete an item before you reach it in the iteration, the item will not be visited at all in the entire loop.
Similarly, if you make changes to a property, it doesn’t guarantee that the item won’t be revisited again. So, if a property is changed it might be visited twice in the loop rather than once.
In addition, if a property is added during iteration, it might or might not be visited during the iteration at all.
Due to these situations, it’s best to avoid making any alterations, deletions, or additions to an object in a for...in
loop.
Here’s an example of adding an element in a for...in
loop. We can see the result of the first loop then the second after making additions in the first loop.
See the Pen Addition in Object Loop by SitePoint (@SitePoint) on CodePen.
As you can see in the above example, the elements that were added weren’t iterated over.
Alternatives to For Loops in JavaScript
So in those situations where a for...in
loop isn’t the best option, what should you use instead? Let’s take a look.
Using a for loop with arrays
It’s never wrong to use a for
loop! The JavaScript for
loop is one of the most basic tools for looping over array elements. The for
loop allows you to take full control of the indices as you iterate an array.
This means that when using the for
loop, you can move forwards and backwards, change items in the array, add items, and more, while still maintaining the order of the array.
The following statement creates a loop that iterates over an array and prints its values to the console.
for (let i = 0; i < arr.length; i++) {
console.log(arr[i]);
}
forEach method for arrays and objects
forEach in JavaScript is a method on array prototypes that allows us to iterate over the elements of an array and their indices in a callback function.
Callback functions are functions that you pass to another method or function to be executed as part of the execution of that method or function. When it comes to forEach
in JavaScript, it means that the callback function will be executed for each iteration receiving the current item in the iteration as a parameter.
For example, the following statement iterates over the variable arr
and prints its values in the console using forEach
:
arr.forEach((value) => console.log(value));
You can also access the index of the array:
arr.forEach((value, index) => console.log(value, index));
JavaScript forEach
loops can also be used to iterate objects by using Object.keys(), passing it the object you want to iterate over, which returns an array of the object’s own properties:
Object.keys(obj).forEach((key) => console.log(obj[key]));
Alternatively, you can forEach
to loop over the values of properties directly if you don’t need access to the properties using Object.values():
Object.values(obj).forEach((value) => console.log(value));
Note that Object.values()
returns the items in the same order as for...in
.
Conclusion
By using the JavaScript for...in
loop, we can loop through keys or properties of an object. It can be useful when iterating object properties or for debugging, but should be avoided when iterating arrays or making changes to the object. I hope you’ve found the above examples and explanations useful.
FAQs about the for loop in JavaScript
for
loop in JavaScript? A for
loop is a control flow statement that repeats a block of code a specified number of times. It consists of an initialization statement, a condition, an increment or decrement statement, and the code to be executed in each iteration.
W
hat are the three parts of afor
loop header? The three parts are:
Initialization statement: Executed once at the beginning.
Condition: Checked before each iteration. If false
, the loop is terminated.
Increment or decrement statement: Executed after each iteration.
for...in
loop differ from a standard for
loop? The for...in
loop is used for iterating over the properties of an object. It assigns the property names to the loop variable. A standard for
loop, on the other hand, is typically used for iterating over arrays or performing a specific number of iterations.
for
and for...of
loops? The for...of
loop is used for iterating over iterable objects (like arrays, strings, etc.) and directly retrieves the values instead of indices. The for
loop, in contrast, is more general and can be used for different types of loops, including those that iterate over indices.
I am a full-stack developer passionate about learning something new every day, then sharing my knowledge with the community.