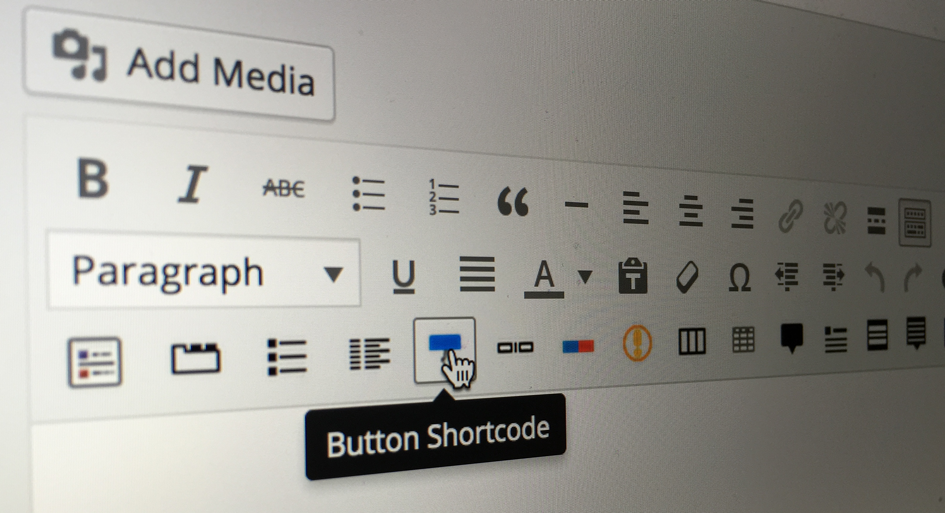
This article is part of a series created in partnership with SiteGround. Thank you for supporting the partners who make SitePoint possible.
Ideally, WordPress authors should never need to edit raw HTML. You should enable plugins and custom meta boxes which allow the user to configure the page as necessary. Unfortunately, there are situations when it’s difficult or impractical to provide UI tools, e.g.:- a widget provides numerous configuration options
- similar widgets can appear multiple times in the page at any location
- widgets can be nested inside each other, such as a button overlaying a video within a sidebar
- a widget’s implementation changes, e.g. you switch from one video hosting platform to another.
[mywidget]
which places an HTML component in the rendered page without the need for coding.
Where to Create Shortcodes
Shortcodes are often created to aid custom plugin usage so you should place those within the plugin code itself. However, you can also place shortcode definitions within a theme’sfunctions.php
file. It’s possibly more practical to create a separate shortcodes.php
file then include that within functions.php
using the statement:
include('shortcodes.php');
Your First “Hello World” Shortcode
A shortcode definition consists:- a function which returns a string of HTML code, and
- a call to the WordPress
add_shortcode()
hook which binds a the shortcode text definition to that function.
<?php
// "Hello World" shortcode
function shortcode_HelloWorld() {
return '<p>Hello World!</p>';
}
add_shortcode('helloworld', 'shortcode_HelloWorld');
(You can omit the <?php
line if one is already present at the top of your file.)
Save the file then enter [helloworld]
somewhere within a page or post. Visit that page and you’ll see it’s been replaced with a “Hello World!” paragraph.
Shortcode Parameters
Shortcodes can have optional parameters, e.g.[sitemap title='Web pages', depth=3]
Parameters are passed as an array to the shortcode function as the first argument. The full code to generate a page hierarchy sitemap:
// sitemap shortcode
function shortcode_GenerateSitemap($params = array()) {
// default parameters
extract(shortcode_atts(array(
'title' => 'Site map',
'id' => 'sitemap',
'depth' => 2
), $params));
// create sitemap
$sitemap = wp_list_pages("title_li=&depth=$depth&sort_column=menu_order&echo=0");
if ($sitemap) {
$sitemap =
($title ? "<h2>$title</h2>" : '') .
'<ul' . ($id? '' : " id="$id"") . ">$sitemap</ul>";
}
return $sitemap;
}
add_shortcode('sitemap', 'shortcode_GenerateSitemap');
The shortcode_atts()
function assigns default values to parameters when required. The PHP extract()
function then converts each array value into the real variables $title
, $id
and $depth
.
(Note array(...)
can be replaced with the shorter [...]
syntax if you are using PHP5.4 or above.)
Add a [sitemap]
shortcode to any post or page and optionally change the parameters, e.g. [sitemap depth=5]
.
Nested BBCode Shortcodes
BBCode (Bulletin Board Code) is a lightweight markup format which, like standard shortcodes, uses [square brackets] to denote commands. This permits shortcodes to contain text content or be nested within each other. Presume your pages require pull quotes and general purpose call-to-action buttons. It would be impractical to create a single shortcode especially when a button could be used on its own or embedded within a quote. We could require HTML such as:<blockquote>
<p>Everything we do is amazing!</p>
<p><a href="/contact-us/" class="cta button main">Call us today</a></p>
</blockquote>
It would could get this wrong when the editor was intimately familiar with HTML. Fortunately, shortcodes provide an easier route, e.g.
[quote]
Everything we do is amazing!
[cta type='main']Call us today[/cta]
[/quote]
The content between the tags is passed to the shortcode function as the second argument. We can create two shortcodes functions:
// [quote] shortcode
function shortcode_Quote($params = array(), $content) {
// default parameters
extract(shortcode_atts(array(
'type' => ''
), $params));
// create quote
return
'<blockquote' .
($type ? " class=\"$type\"" : '') .
'>' .
do_shortcode($content) .
'</blockquote>';
}
add_shortcode('quote', 'shortcode_Quote');
// [cta] shortcode
function shortcode_CallToAction($params = array(), $content) {
// default parameters
extract(shortcode_atts(array(
'href' => '/contact-us/',
'type' => ''
), $params));
// create link in button style
return
'<a class="cta button' .
($type ? " $type" : '') .
'">' .
do_shortcode($content) .
'</a>';
}
add_shortcode('cta', 'shortcode_CallToAction');
Note the use of do_shortcode($content)
function which applies further shortcodes to the content when they exist.
Shortcodes are easy to implement and can be changed or enhanced quickly. I recommend creating a shortcode cheatsheet with examples so editors have a reference when complex functionality is required.
If you’re looking for somewhere to host your WordPress site after you’ve got your shortcodes all figured out, take a look at our partner, SiteGround. They offer managed WordPress hosting, with one-click installation, staging environments, a WP-CLI interface, pre-installed Git, autoupdates, and more!
Frequently Asked Questions about Creating Custom WordPress Shortcodes
What are the benefits of using WordPress shortcodes?
WordPress shortcodes offer a convenient way to add complex content to your posts or pages without having to write extensive HTML or PHP code. They are essentially shortcuts that allow you to embed files or create objects that would normally require lots of complicated, ugly code in just one line. This makes your content easier to manage and more user-friendly. Shortcodes can be used to add a variety of features such as forms, sliders, videos, and more.
How can I create a basic shortcode in WordPress?
To create a basic shortcode in WordPress, you need to add a function to your theme’s functions.php file. This function should define what the shortcode does when it is called. Here is a simple example:function my_shortcode() {
return 'Hello, World!';
}
add_shortcode('greeting', 'my_shortcode');
In this example, the shortcode [greeting] will output “Hello, World!” when added to a post or page.
Can I pass parameters to a WordPress shortcode?
Yes, you can pass parameters to a WordPress shortcode. This allows you to customize the output of the shortcode based on the parameters provided. Here is an example:function my_shortcode($atts) {
return 'Hello, ' . $atts['name'] . '!';
}
add_shortcode('greeting', 'my_shortcode');
In this example, the shortcode [greeting name=”John”] will output “Hello, John!”.
How can I create a shortcode that wraps content?
To create a shortcode that wraps content, you need to add a function to your theme’s functions.php file that takes an additional parameter for the content. Here is an example:function my_shortcode($atts, $content = null) {
return '<div class="my-class">' . $content . '</div>';
}
add_shortcode('myshortcode', 'my_shortcode');
In this example, the shortcode [myshortcode]…[/myshortcode] will wrap the content in a div with the class “my-class”.
Can I use shortcodes in widgets?
Yes, you can use shortcodes in widgets. However, by default, WordPress text widgets do not process shortcodes. To enable this functionality, you need to add the following line of code to your theme’s functions.php file:add_filter('widget_text', 'do_shortcode');
This tells WordPress to process shortcodes in text widgets.
How can I create a shortcode that outputs dynamic content?
To create a shortcode that outputs dynamic content, you need to add a function to your theme’s functions.php file that generates the dynamic content. Here is an example:function my_shortcode() {
return date('Y-m-d H:i:s');
}
add_shortcode('currentdatetime', 'my_shortcode');
In this example, the shortcode [currentdatetime] will output the current date and time.
Can I nest shortcodes?
Yes, you can nest shortcodes. This means you can use one shortcode inside another. However, not all shortcodes are designed to be nested, and doing so may cause unexpected results. Always test nested shortcodes thoroughly to ensure they work as expected.
How can I create a shortcode that outputs a form?
To create a shortcode that outputs a form, you need to add a function to your theme’s functions.php file that generates the form HTML. Here is an example:function my_shortcode() {
return '<form action="/action_page.php">
First name:<br>
<input type="text" name="firstname" value="Mickey"><br>
Last name:<br>
<input type="text" name="lastname" value="Mouse"><br><br>
<input type="submit" value="Submit">
</form> ';
}
add_shortcode('myform', 'my_shortcode');
In this example, the shortcode [myform] will output a simple form.
Can I use shortcodes in template files?
Yes, you can use shortcodes in template files. To do this, you need to use the do_shortcode function. Here is an example:echo do_shortcode('[myshortcode]');
This will output the result of the [myshortcode] shortcode.
How can I debug a shortcode that is not working?
If a shortcode is not working, there are several steps you can take to debug it. First, check the syntax of the shortcode to make sure it is correct. Second, check the function that the shortcode calls to make sure it is defined and working correctly. Third, check for conflicts with other plugins or themes that may be causing the shortcode to not work. If all else fails, you can use WordPress’s built-in debugging tools to get more information about what is going wrong.
Craig is a freelance UK web consultant who built his first page for IE2.0 in 1995. Since that time he's been advocating standards, accessibility, and best-practice HTML5 techniques. He's created enterprise specifications, websites and online applications for companies and organisations including the UK Parliament, the European Parliament, the Department of Energy & Climate Change, Microsoft, and more. He's written more than 1,000 articles for SitePoint and you can find him @craigbuckler.