In the beginning, there was HTML — and everyone was happy. Then, one day, someone clever decided that they needed to have dynamic content on their Website, invented a scripting language that generated HTML, and all hell broke loose. Within months, Web pages had grown into a jungle of HTML, scripting code, and SQL statements.
At first, Java’s take on all this was the Servlet. Although useful in certain circumstances, it proved cumbersome and unwieldy. The saviour was to come in the form of Java ServerPages. Able to leap across buildings in a single bound, JSPs looked like a scripting language and acted like scripting language, but in actual fact were nothing more than a clever way of writing Servlets! JSPs solved a lot of problems that were inherent within Servlets, but they introduced a number of issues as well.
Pages could easily become a mess of scriptlet code and HTML fragments. Worse still, JSPs allowed you to avoid having to write JavaBeans to take care of your business logic; putting all your site functionality into the Web page itself was a quick fix that, more often than not, would come back and bite you when you least expected it. Finally, the typical front end developer took to JSP scripts like a fish to a bicycle, shying away from the complexities of Vectors, Request objects and null pointer exceptions in favour of simpler pleasures.
Why am I telling you all this? Because, just when it seemed like everything was headed in a downward spiral, there came a solution! Enter stage left, the Java Standard Tag Library.
Meet the JSTL
The Java Standard Tag Library (or JSTL) is nothing more than a Java Tag Library that provides us with a number of Standard functions. Ok, ok — put another way, the JSTL is going to make your quagmire of JSP script and HTML look like regular plain old HTML pages again. And that is a good thing. Your pages will be more readable (no more scriptlets), your code architecture will become a lot cleaner (no more HTML in your JavaBean classes) and, best of all, the front end developers you work with will invite you to the pub at lunchtime like they used to.
Ok, just to whet your appetite before we talk about how to get the thing installed, here’s a fairly typical piece of code you might see in a JSP page:
<% For (Enumeration e = shoppingCart.getProducts();
e.hasMoreElements();) {
Product thisProduct = (Product)e.nextElement();
%>
<br /><%=thisProduct.getDescription()%>
<% } %>
Clear as mud, right? Here’s the same functionality written using the JSTL:
<c:forEach var="thisProduct" items="${shoppingCart.products}">
<br /><c:out value="${thisProduct.description}"/>
</c:forEach>
By now, the HTML developers you work with aren’t just inviting you to the pub, they’re getting the rounds in as well!
Ok, let’s get this thing installed. You will need an application server that adheres to the JSP 1.2 spec. For most people that means Tomcat 4.0+. Once Tomcat is installed, you’ll need to download a copy of the JSTL itself. You can get hold of a copy from the Jakarta Apache site (see Resources at the end of this article). Download the code for this article here.
- Create a new Web application in Tomcat (you can just create a new directory under the webapps/ directory). For this article, we’ll call the Web application “jstl_stuff”.
- Unpack the JSTL archive.
- Create a “WEB-INF” directory within “jstl_stuff”, and a “lib” directory within “WEB-INF”.
- Copy everything from the lib directory of your JSTL archive into <Tomcat Home>/webapps/jstl_stuff/WEB-INF/lib.
- Restart Tomcat.
To test the installation, create a page entitled index.jsp in the /jstl_stuff directory, and add the following code:
<%@ taglib prefix="c" uri="http://java.sun.com/jstl/core" %>
<c:out value="Doh! It's working!"/>
Now try browsing to http://localhost:8080/jstl_stuff. If you see a page that displays “Doh! It’s working!” and nothing else, everything is working perfectly. If you see an explosion of java exceptions, something has gone wrong, and it’s time to start checking your Tomcat configuration.
The Components Explained
I’m going to be referencing the following class (simpsons.Homer
) for the examples. You can compile it and place it in <tomcat home>/webapps/WEB-INF/classes/simpsons, or you could write your own.
package simpsons;
import java.util.Vector;
public class Homer {
private int iq;
private Vector braincells;
public Homer() {
iq = 24;
braincells = new Vector();
braincells.add("Eating");
braincells.add("Drinking");
braincells.add("Sleeping");
}
public int getIq() {
return iq;
}
public void setIq(int iq) {
if (iq < 100) {
this.iq = iq;
}
}
public Vector getBraincells() {
return braincells;
}
}
Note: in the following examples, we need to declare that we’re going to use the Homer
class in our JSP pages. Remember to add the following line to the top of your JSP pages after you’ve compiled the class:
<jsp:useBean id="homer" class="simpsons.Homer" scope="page" />
The JSTL is split into 4 components:
- Core – The main tags used within the library
- XML – A set of XML processing tags
- Format – Internationalization and formatting tags
- SQL – Tags for accessing an SQL Database
We are going to cover the Core and Format set of tags in this article. If you want to get to grips with either of the others, see the resource links at the end of this article.
In order to inform Tomcat that we are about to use the JSTL core tags in our page, we need to include the following taglib
directive in every page in which JSTL tags are used:
<%@ taglib prefix="c" uri="http://java.sun.com/jstl/core" %>
As I mentioned earlier, the idea behind the JSTL is to provide a basic level of functionality to the programmer, so that JSP scriptlets are no longer needed. For example, if we want to assign a value to a variable, we can use the set
action:
<c:set var="homer" value="Doh!" />
To output something to HTML, we can use the out
action:
<c:set var="homer" value="Doh!" />
<c:out value="${homer}" />
Running this should provide us with a single “Doh!”
You can set the property of a JavaBean as well. For example, if wanted to set homer
‘s IQ property we could do:
<c:set target="${homer}" property="iq" value="34"/>
Veteran JSP coders will probably be scratching their heads at this point. This part of code might look unusual:
${homer}
Welcome to the JSTL Expression Language (or EL)! The EL is a method of accessing Java variables in a much simpler way than the old “JSP-style” involved. The EL is both simple and powerful. Using it, we can access the variables of an object in a wide variety of ways:
${homer.iq}
The above would access the homer object’s iq
property.
${homer.braincell[1]}
The above would return the second element of the homer
object’s braincell collection. Note that we don’t need to know (and, indeed, don’t care) whether the braincell property of the homer
object is an array or a Vector (or anything else that implements the List interface); the EL does all the work for us.
We can also use the EL to evaluate expressions. So, for example, the following would evaluate to true:
${homer.iq < 50}
We can now start to use the EL and the JSTL tags to radically simplify our JSP pages.
Conditional and Iterative Operations
Most of the time, JSP scriptlets are used to perform conditional (if..else) or iterative (for, while) tasks. The JSTL core tags provide this functionality without having to resort to scriptlets. The <c:if ...>
and <c:choose ...>
tags provide the basic conditional operations. For example, a simple if statement would read:
<c:if test="${homer.iq < 50}">
Doh! My IQ is <c:out value="${homer.iq}"/>
</c:if>
To perform an if…else operation we can use the <c:choose>
tag, like this:
<c:choose>
<c:when test="${homer.iq < 50}">
Doh!
</c:when>
<c:when test="${homer.iq > 50}">
Wohoo!
</c:when>
<c:otherwise>
An IQ of 50? Wohoo!
</c:otherwise>
</c:choose>
Looping can be performed using the <c:forEach ...>
tag:
<c:forEach var="braincell" items="${homer.braincells}">
<br /><c:out value="${braincell}"/>
</c:forEach> /#epc#/
This will display:
Eating
Drinking
Sleeping
The forEach
tag provides us some optional attributes. For example, we can write:
<c:forEach items="${homer.braincells}" var="braincell" begin="1" end="2" varStatus="status">
<br />Braincell <c:out value="${status.count}"/>: <c:out value="${braincell}" />
</c:forEach>
The above will display:
Braincell 1: Drinking
Braincell 2: Sleeping
Here we’ve used the begin
and end
parameters to define where the loop will start and terminate. We also use the varStatus
object. This object provides an interface into the state of the loop. Check the resources at the end for further information.
JSTL and URL Generation
The JSTL also provides tags to help us tidy up URL generation. Scriptlet code is often used to construct URLs that pass parameters to other pages, and can get messy very quickly. With the JSTL we can construct URLs in a much neater fashion. For example:
<c:url var="thisURL" value="homer.jsp">
<c:param name="iq" value="${homer.iq}"/>
<c:param name="checkAgainst" value="marge simpson"/>
</c:url>
<a href="<c:out value="${thisURL}"/>">Next</a>
The above would generate a URL that reads:
homer.jsp?iq=24&checkAgainst=marge+simpson
Notice how the JSTL has encoded the checkAgainst
parameter for use in the URL, replacing the space with a +
. No more worrying about invalid URLs! One extra bonus of using the url
tag is to aid those surfing without session cookies enabled in their browser. The JSTL will check and automatically add a sessionId
to the link if session cookies have been disabled.
Finally, a quick mention of the format tags. These are separate from the core tags, and have to be imported with the statement thus:
<%@ taglib prefix="fmt" uri="http://java.sun.com/jstl/fmt" %>
The above appears at the top of the JSP page. The format tags provide powerful text formatting functions. Just as a brief experiment, try running the following in a JSP page:
<fmt:formatNumber value="34.5423432426236" maxFractionDigits="5" minFractionDigits="3"/>
<fmt:formatNumber value="34.5423432426236" type="currency"/>
<fmt:parseDate value="2/5/53" pattern="dd/MM/yy" var="homersBirthday"/>
<fmt:formatDate value="${homersBirthday}" dateStyle="full"/>
The above example parses a date and then returns the date in a more verbose style. We can even add locale specifics to date formatting, for example:
<fmt:timeZone value="US/Eastern">
<fmt:parseDate value="2/5/53" pattern="dd/MM/yy" var="homersBirthday"/>
<fmt:formatDate value="${homersBirthday}" dateStyle="short"/>
</fmt:timeZone>
Would output:
5/2/53
What’s the Big Deal?
As you can see, the JSTL is a large topic. We have only covered a small part of the core and format libraries here; there are also libraries for dealing with XML as well as a set of tags for JDBC access.
By now you’re probably thinking one of two things; either you’re thinking, “That’s great, my pages will stop looking like a mess of scriptlets,” or, “What’s the point? I have to learn all this stuff and I don’t really get any extra functionality.”
As I mentioned at the start of this article, the JSTL is really all about making your life easier in the long run. Using the JSTL helps in a number of areas:
- It helps us separate business logic (into JavaBeans) from presentation logic (into JSTL tags).
When you start using the JSTL you will notice it enforces you to write better, cleaner code. It’s a subtle change at first, but as you get more comfortable with the tags, you’ll find it IS faster than developing with scriptlets.
There is a fourth reason for looking into using JSTL in your own projects, and it’s a biggie: Java Server Faces. Sometime next year (2004) will see the arrival of application servers that conform to the JSP 2.0 specification, allowing developers to start using Sun’s much-ballyhooed Java Server Faces framework. It’s an exciting development, and one that JSTL developers will have a head start on; the JSF will make heavy use of the Expression Language as well as the JSTL tags themselves. Making use of the JSTL now will not only help with your projects today, it’ll give you a head start when the JSF servers start coming out in 2004.
Resources
A supremely useful reference is Sun’s JSTL specification PDF, which you can find at:
http://jcp.org/aboutJava/communityprocess/final/jsr052/
It is in PDF format and makes an excellent reference.
The Sun JSTL area is at:
http://java.sun.com/products/jsp/jstl/
A good three part primer at IBM developerWorks:
http://www-106.ibm.com/developerworks/java/library/j-jstl0211.html
The Jakarta JSTL site:
http://jakarta.apache.org/taglibs/doc/standard-1.0-doc/intro.html
Downloads
The Jakarta Implementation can be downloaded at:
http://apache.mirrors.ilisys.com.au/jakarta/taglibs/standard-1.0/binaries/jakarta-taglibs-standard-1.0.4.zip
Tomcat can be downloaded here:
http://apache.planetmirror.com.au/dist/jakarta/tomcat-4/tomcat-4.1.27.zip
Frequently Asked Questions (FAQs) about Java Standard Tag Library (JSTL)
What is the main purpose of the Java Standard Tag Library (JSTL)?
The Java Standard Tag Library (JSTL) is a crucial component of JavaServer Pages (JSP). It provides a collection of tags that simplify the JSP development process. These tags encapsulate core functionalities common to many JSP applications, such as iteration, conditionals, XML manipulation, internationalization, and SQL. Instead of mixing tags with script elements, JSTL allows developers to write JSP pages using tags, which are more readable and maintainable.
How does JSTL differ from traditional scripting in JSP?
Traditional scripting in JSP involves the use of scriptlets, which are Java code snippets embedded in HTML code. This can lead to unmanageable and hard-to-read code. JSTL, on the other hand, provides a collection of custom tags that can be used to control the flow of execution, manipulate XML documents, access databases, and perform other common tasks. This makes the code cleaner, easier to read, and maintain.
How can I install and use JSTL in my JSP application?
To use JSTL in your JSP application, you first need to download the JSTL library and add it to your project’s classpath. Then, you can use the taglib directive at the beginning of your JSP page to include the JSTL core library. This will allow you to use JSTL tags in your JSP pages.
What are the different types of JSTL tags?
JSTL provides four types of tags: Core, Formatting, SQL, and XML. Core tags provide flow control, variable support, URL management, and other utilities. Formatting tags help with formatting of numbers, dates, and i18n support. SQL tags are for interaction with databases, and XML tags are for XML processing.
Can I create my own custom tags in JSTL?
Yes, JSTL allows developers to create their own custom tags. This can be useful when you need to encapsulate complex logic into a reusable component. To create a custom tag, you need to create a tag handler class and a tag library descriptor file.
How can I use JSTL tags for database access?
JSTL provides SQL tags that can be used to interact with databases. These tags allow you to execute SQL queries and process the result sets directly in your JSP pages. However, it’s important to note that for complex applications, it’s recommended to use a more robust data access method, such as JDBC or Hibernate.
How can I handle exceptions in JSTL?
JSTL provides the c:catch tag for exception handling. This tag can be used to catch and handle any exceptions thrown within its body. The caught exception can then be accessed using the var attribute of the c:catch tag.
How can I use JSTL for XML processing?
JSTL provides a set of XML tags that can be used for parsing and manipulating XML documents. These tags support XPath expressions and can be used to iterate over XML nodes, transform XML documents using XSLT, and more.
How can I use JSTL for internationalization (i18n)?
JSTL provides a set of tags for internationalization (i18n). These tags can be used to format dates, numbers, and messages according to a specific locale. This makes it easier to develop applications that support multiple languages and regions.
Can I use JSTL with other Java frameworks like Spring?
Yes, JSTL can be used with other Java frameworks like Spring. In fact, Spring MVC provides built-in support for JSTL. This allows you to use JSTL tags in your Spring views, making them more readable and maintainable.
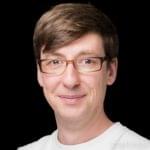
Ben founded London agency Solid State Group and The Hoxton Mix. He’s passionate about coding, starting companies, funding companies and using tech to make the world more enjoyable. He wrote his first web page in 1994, which probably makes him too old.