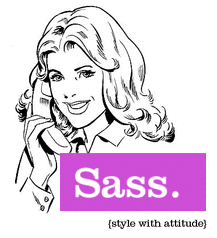
Sass, (Syntactically Awesome Stylesheets) is a meta-language, which has redefined CSS for “programmers” around the world.
Not only does it supplement CSS, it brings out the fun in web designing. Apart from the weird logo of the “sassy” lady attending to the phone, Sass is truly elegant and awesome (but that could just be me).
I remember my colleague who approached CSS from a programming background. He complained that it lacked certain functions, common to all traditional programming languages. As you probably know, CSS has nothing to do with that kind of programming. However, dynamic style-sheet languages, such as
LESS and
Sass, offer many additional features over CSS that mimic traditional programming constructs.
You might have noticed something like this in the Gemfile of your web app (if you’re running Rails 3.1)
[gist id=”2691554″]
Rails 3.1+ provides support for Sass by default, which has become popular among the Rails community.
Until Rails 3.0, the asset pipeline was available only via third-party libraries such as Sprockets. The assets (images, JavaScript and stylesheet files) were placed under the public directory. In Rails 3.1, you can place them under apps/assets.
[gist id=”2691563″]
Rails treats your javascript code, stylesheets and images as assets and helps you boost the speed in a development environment. The asset pipeline offers you the ability to precompile, concatenate and compress your assets.
Fingerprinting, which is hardwired into the asset pipeline, provides a reliable caching technique.
So, What is the Asset Pipeline?
The asset pipeline enables you to store your assets in one central library. Technically speaking, it links together your assets into one master file, reducing the number of requests made by the browser. This can greatly speed up page load time. (I will get back to this soon)
It also allows you to write your assets in dynamic languages, such as SASS for CSS or Coffeescript for JavaScript. As a rails developer, you may feel more comfortable with Sass and CoffeeScript, but you can still use the “base” languages to create your assets.
You can also place your assets under
- lib/assets – assets for libraries, such as jQuery.
- vendor/assets – assets associated with third party apps and frameworks, such as plupload.
To get a better idea about the asset pipeline, visit the official
Ruby on Rails guide.
Now, let’s dig a bit deeper by exploring the stylesheet assets of our new rails application.
[gist id=”2691568″]
Open up app/assets/stylesheets/application.css (which is the default manifest file for stylesheets) in your favorite text editor, and you will find the following CSS comment:
[gist id=”2691569″]
Although the whole text is a CSS comment, the lines
[gist id=”2691571″]
are utilized by Sprockets to include the required stylesheets, and to concatenate them into one master file.
[gist id=”2691573″]
This ensures that all the CSS/SCSS files in the current directory (including any sub directories) are included in the web app’s CSS.
[gist id=”2691577″]
has also been specified to ensure that the application.css file is included.
To work its magic, all we need to do is create a new file. Lets name it sass_sample.css.scss. You can place your CSS in several files since they will get concatenated at run time, as long as they are under the stylesheets directory.
The extension SCSS stands for sassy CSS and it’s 100% compatible with CSS3. All your CSS3 code will work without any trouble. Sass has another indented syntax with the extension .sass, but it’s old and has lost ground in the community.
SASS is beautiful, but wouldn’t it be worth more if we could somehow integrate SASS with a CSS framework? Blueprint and 960 Grid are awesome tools and here’s how you can integrate them with SASS. Since Blueprint and 960 Grid are 3rd party frameworks, we can place them under /vendor/assets/stylesheets.
For the sake of this tutorial, I will guide you through the process of setting up Blueprint with SASS.
- Download the latest Blueprint CSS tarball from blueprint.org or GitHub.
- Open up the command line and untar the files.
[gist id=”2691591″]
- Copy the blueprint folder to your app’s vendor/assets/stylesheets/.
[gist id=”2691599″]
- Add these lines to /app/views/layouts/application.html.erb. This will link the blueprint stylesheet to our web app’s layout.
[gist id=”2691626″]
Make sure they are placed at the top, before application.css gets linked. That’s it, you are done with setting up blueprint with SASS.
Although Blueprint is a pure CSS framework, it will work out with SASS because SASS’s new .scss syntax is completely compatible with CSS. Can you believe that? You can now access Blueprint’s reset, typography, grid, forms, ie, and print stylesheets in your web app without any hassle.
If you are a twitter bootstrap fan, there is also a gem for that.
[gist id=”2691630″]
But you would have to add the line
[gist id=”2691632″]
into your .scss file to make it work.
Enough with the talk, let’s start coding. If you’re like me, you like throwing around the same colors or gradients on the page with some slight variation. The hardest part is remembering them all, but SASS lets you store colors as variables. Wouldn’t it make more sense to use $androidgreen rather than #A4C639?
[gist id=”2691640″]
There is another interesting technique available in SASS; it lets you do arithmetic operations with variables. You can add two colors like so:
[gist id=”2691648″]
SASS can do much more than just simple color manipulation; you can also manipulate HSL values of colors and play around with alpha transparency. These functions let you play god in the world of web designing.
In addition to color manipulation, you can also nest your CSS code.
[gist id=”2691654″]
It gets compiled into;
[gist id=”2691661″]
Sass offers several more useful functions over CSS, such as mixins, selector inheritance and control structures which allow developers to create static stylesheets whilst making use of dynamic methods. SASS also does a good job in keeping your code “DRY” and making it easier to maintain.
If that sounds cool, stay tuned for the next post where a lot more will be covered on SASS and you will start coding in SCSS.
Frequently Asked Questions (FAQs) about SASS in Rails
What is the difference between SASS and SCSS in Rails?
SASS (Syntactically Awesome Stylesheets) and SCSS (Sassy CSS) are both preprocessor scripting languages that are interpreted into Cascading Style Sheets (CSS). The key difference between the two lies in their syntax. SASS follows an indentation-based syntax, meaning it doesn’t use semicolons or brackets, while SCSS syntax is similar to CSS, using brackets and semicolons. In Rails, both can be used interchangeably, and Rails will compile both to CSS.
How do I install SASS in Rails?
To install SASS in Rails, you need to add the ‘sass-rails’ gem to your Gemfile. This gem is usually included by default in a new Rails application. If it’s not, you can add it manually by including the line ‘gem ‘sass-rails’, ‘~> 5.0’ in your Gemfile. After adding the gem, run ‘bundle install’ to install it.
How do I convert CSS to SASS in Rails?
Converting CSS to SASS in Rails is straightforward. Rename your .css file to .scss or .sass, depending on the syntax you prefer. Then, you can start converting your CSS code to SASS. Rails will automatically compile your SASS code into CSS.
How do I use variables in SASS?
In SASS, you can define variables to store values that you want to reuse throughout your stylesheet. To define a variable, use the ‘ symbol followed by the variable name. For example, ‘$primary-color: #333;’. You can then use this variable anywhere in your stylesheet by referencing its name, like ‘color: $primary-color;’.
How do I use mixins in SASS?
Mixins in SASS are a way of including (“mixing in”) a bunch of properties from one rule-set into another rule-set. They are defined using the ‘@mixin’ directive. For example, ‘@mixin border-radius($radius) { -webkit-border-radius: $radius; -moz-border-radius: $radius; -ms-border-radius: $radius; border-radius: $radius; }’. You can then include the mixin in your code using the ‘@include’ directive, like ‘@include border-radius(10px);’.
How do I use nesting in SASS?
Nesting in SASS allows you to nest your CSS selectors in a way that follows the same visual hierarchy of your HTML. For example, ‘.container { h1 { color: blue; } p { color: red; } }’. This will compile to ‘.container h1 { color: blue; } .container p { color: red; }’ in CSS.
How do I import files in SASS?
You can import files in SASS using the ‘@import’ directive. This allows you to split your SASS code into smaller, more manageable files. For example, ‘@import ‘variables’;’ will import the ‘_variables.scss’ file.
How do I use operators in SASS?
SASS supports standard math operators like +, -, *, /, and %. For example, ‘p { margin: $var * 1.5; }’. This will multiply the value of ‘$var’ by 1.5 and use it as the margin.
How do I use control directives in SASS?
Control directives are scripting constructs that let you use logic in your SASS code. They include @if, @for, @each, and @while. For example, ‘@each $name in a, b, c { .#{$name} { width: 2em * $name; } }’. This will output three classes, ‘.a’, ‘.b’, and ‘.c’, each with a different width.
How do I use functions in SASS?
Functions in SASS allow you to define complex operations on SASS values that you can reuse throughout your stylesheet. They are defined using the ‘@function’ directive. For example, ‘@function double($n) { @return $n * 2; }’. You can then use this function in your code like ‘width: double(5px);’.