- Why Not Use a WordPress Plugin?
- What You’ll Need
- Getting jQuery Colorbox Into Your Theme’s Files
- Integrating jQuery Colorbox Into Your Theme
- Creating the Main JavaScript File
- Writing the jQuery Script
- Moving on to PHP
- Internationalizing the JQuery Colorbox Text Strings
- Conclusion
- Frequently Asked Questions (FAQs) about Adding a Stylish Lightbox Effect to the WordPress Gallery
WordPress comes with built-in gallery functionality. Adding a gallery to a post or page involves performing a few simple steps:
- Click the Add Media button
- Click the Create Gallery link
- Select your images by clicking on them
- Enter at least the Title and Alt text into the appropriate textbox and click the Create a new gallery button
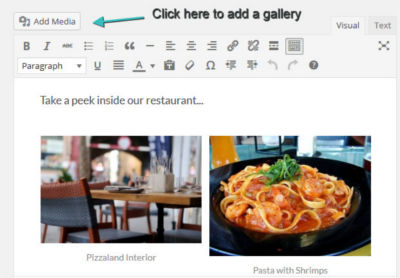
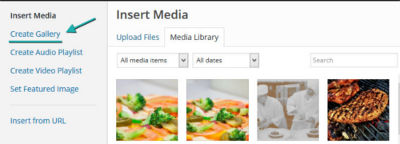
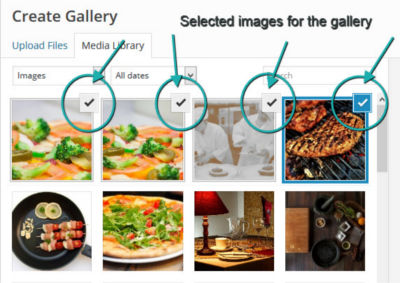
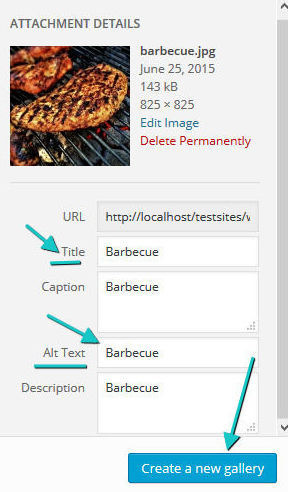
What WordPress doesn’t offer out of the box is the ability to zoom in on each gallery image with a cool lightbox effect.
In fact, you can adjust the gallery settings so that images link to a dedicated page (i.e., attachment page), to the image file itself, or to nothing at all.
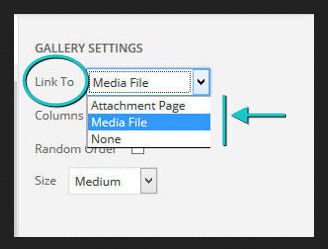
In this post, I’m going to show how to integrate the popular jQuery Colorbox plugin by Jack Moore into the native WordPress gallery.
As a result, clicking an image will cause it to pop up with a smooth animation effect. Visitors will be able to read image captions and navigate through the images without leaving the gallery page.
The finished product will be something looking like this.
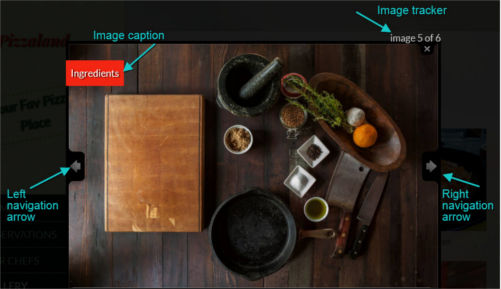
Why Not Use a WordPress Plugin?
At this point, I can hear you say: “There are plenty of WordPress plugins that make it a snap to add galleries and lightbox functionality to a WordPress site. jQuery Colorbox itself has a number of implementations as a WordPress plugin. Why should I bother coding this functionality myself?”
For one thing, here we’re considering a very specific piece of functionality, i.e., adding lightbox effect just to the WordPress gallery. Most plugins have a lot more to offer, and with it also comes a quantity of extra code. If you’re looking for high flexibility like adding lightbox effects to images in posts and pages, to custom links, or to other kinds of content, adjusting plugin’s settings from the admin panel, etc., then go ahead and take the WP plugin route. However, if your goal is to accomplish a very focused task, as it’s the case right now, running a WP plugin is probably an overkill.
Another reason could simply be personal preference. For myself, I’d rather use WP plugins for back-end tasks like sending a contact form, managing a shopping cart, adding membership or forum functionality, etc. For most tasks related to front-end development, e.g. styling site elements, creating animations, implementing jQuery sliders and lightbox, I opt for the do-it-yourself approach.
Finally, coding is fun, educational (there’s always something new to learn along the way), and in this case it won’t take a lot of your time.
What You’ll Need
To follow along, you’ll need a WordPress installation, preferably on a local server, and a theme that provides CSS styles for the native WordPress gallery. For the purposes of this tutorial, a child theme of any of the themes bundled with WordPress will be fine.
It’s time to dive in!
Getting jQuery Colorbox Into Your Theme’s Files
First things first: download a copy of jQuery Colorbox and unzip the compressed file somewhere in your hard drive.
The files you need to make jQuery Colorbox work are the following:
jquery.colorbox-min.js
;colorbox.css
and theimages
folder from one of the five Colorbox skins. These can be found in the folders calledexample1
,example2
, etc, through toexample5
. Try each skin out in your browser and pick the one you like best. For this tutorial, I chose the skin contained in theexample3
folder.
Next, to keep the project well organized, add a folder to your theme’s files and call it colorbox
. Drop the files listed above inside this folder.
jQuery Colorbox comes with its text strings already translated into an impressive number of languages. The translations are located inside the i18n
folder. There’s no need for you to copy this folder into your project, but it’s important to be aware of it. It’s a great resource if you intend to provide translations for your theme.
Integrating jQuery Colorbox Into Your Theme
Integrating Colorbox into your theme involves a series of steps. Some of these steps deal with the jQuery code necessary to make Colorbox do its job. Another series of steps handles the task of making WordPress ‘aware’ of Colorbox and involves writing PHP code.
Let’s get to work!
Creating the Main JavaScript File
Colorbox is a jQuery plugin, therefore you need to create a JavaScript file that will contain the necessary initialization and configuration code.
Add a new folder to the root of your theme and call it js
. Next, inside the js
folder, create a new file and call it main.js
(or any other name you prefer for your theme’s main JavaScript file).
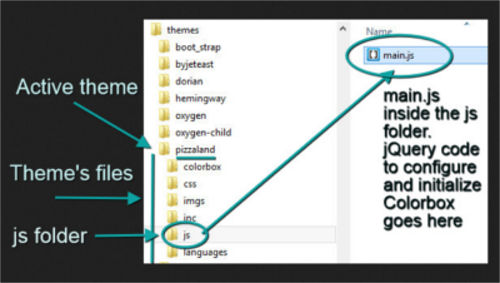
Open main.js
and enter a jQuery no conflict wrapper to make sure the jQuery $
sign doesn’t conflict with other scripts using the same alias symbol:
(function($) {
//All your code goes inside this wrapper
})(jQuery);
Now your jQuery document has been set up. Let’s keep going!
Writing the jQuery Script
Initializing jQuery Colorbox is as easy as writing the following line of code:
selector.colorbox();
selector stands for the HTML anchor element on which you want to apply the lightbox effect.
But, with such a highly configurable plugin, you can do a bit more. There are plenty of settings you can adjust to make Colorbox do what you want. Let’s start by tweaking some of them. Inside the jQuery no conflict wrapper, write the following code snippet:
//Settings for lightbox
var cbSettings = {
rel: 'cboxElement',
width: '95%',
height: 'auto',
maxWidth: '660',
maxHeight: 'auto',
title: function() {
return $(this).find('img').attr('alt');
}
}
The code above stores a bunch of settings inside a JavaScript object literal called cbSettings
:
- rel is used to group photos together. Setting this attribute enables the navigation arrows in the lightbox so that users can move from one photo to the previous or next photo in the group. cboxElement is a class that Colorbox automatically adds to the anchor links where lightbox functionality is attached, but you’re free to group photos by a different name.
- width and height refer to the lightbox dimensions. A percentage value for the width helps to keep the component fluid and responsive. maxWidth and maxHeight refer to the maximum width and maximum height of the content loaded in the lightbox, which in this case is the gallery photo.
- title can be used to display a caption for the photo in the lightbox. Here it’s set to a short function that tells Colorbox to extract the value of the image’s alt attribute and use it as image caption.
You can find a list of all the available settings on the jQuery Colorbox website.
Once the settings are configured, initialize Colorbox:
//Initialize jQuery Colorbox
$('.gallery a[href$=".jpg"],
.gallery a[href$=".jpeg"],
.gallery a[href$=".png"],
.gallery a[href$=".gif"]').colorbox(cbSettings);
The snippet above selects all the anchor tags inside the element with a class of gallery
. Further, the selected anchor tags must have a href
value that ends in .jpg
, .jpeg
, .png
, or .gif
(the most common image file formats). Finally, Colorbox is called on this selection passing in the cbSettings
object as an argument.
Contemporary web design is responsive web design, therefore it’s important for us that the lightbox should adapt to all screen sizes. In view of this, add the following snippet that takes care of this crucial aspect:
//Keep lightbox responsive on screen resize
$(window).on('resize', function() {
$.colorbox.resize({
width: window.innerWidth > parseInt(cbSettings.maxWidth) ? cbSettings.maxWidth : cbSettings.width
});
});
On browser resize, the $.colorbox.resize()
method is triggered. Inside this method, the code above sets the width
value to a JavaScript conditional ternary operator statement. This statement does the following:
- it retrieves the screen’s
width
; - it compares this
width
with themaxWidth
value from the plugin’s settings, which in our case it’s660px
; - if the screen’s
width
is bigger than660px
, it adjusts the lightboxwidth
to the value of660px
. However, if the screen’s size is smaller than660px
, the lightboxwidth
is resized to thewidth
stated in the settings, which in this case is95%
.
More on this solution is available on the Colorbox GitHub page.
That’s it for now on the JavaScript front, you’ll come back to it later to deal with WordPress internationalization. Let’s tackle the WordPress side of the project.
Moving on to PHP
At this time, WordPress knows nothing of the presence of jQuery Colorbox in your theme. Neither the plugin’s files nor main.js
are being loaded. The recommended practice for loading stylesheets and JavaScript files in WordPress is by using wp_enqueue_style() and wp_enqueue_script() respectively.
Find functions.php
in your theme’s files and open it in a code editor. It’s likely your theme already has a function to enqueue style.css
. If you can’t find it, adapt the function in the code snippet below to your theme.
function themeslug_enqueue_styles_scripts() {
//Colorbox stylesheet
wp_enqueue_style( 'colorbox', get_template_directory_uri() .
'/colorbox/colorbox.css' );
//Your theme CSS
wp_enqueue_style( 'themeslug-style', get_stylesheet_uri() );
//Colorbox jQuery plugin js file
wp_enqueue_script( 'colorbox', get_template_directory_uri() .
'/colorbox/jquery.colorbox-min.js', array( 'jquery' ), '', true );
//Add main.js file
wp_enqueue_script( 'themeslug-script', get_template_directory_uri() .
'/js/main.js', array( 'colorbox' ), '', true );
}
//Hook the function above
add_action( 'wp_enqueue_scripts', 'themeslug_enqueue_styles_scripts' );
Let’s explain what the code above does.
- It wraps all the calls to
wp_enqueue_style()
andwp_enqueue_script()
inside a function calledthemeslug_enqueue_styles_scripts()
. - Inside
themeslug_enqueue_styles_scripts()
, the Colorbox CSS file is enqueued before the theme’s stylesheet. - The Colorbox JavaScript file is enqueued pointing to the jQuery library as a dependency, i.e.,
jquery.colorbox-min.js
loads only after the jQuery library file has already been loaded. - The theme’s JavaScript file,
main.js
, is enqueued next, indicatingjquery.colorbox-min.js
as a dependency. - The argument
true
at the end ofwp_enqueue_script()
ensures that the JavaScript files are loaded in the page’sfooter
area, before the closing</body>
tag. - Finally, the
themeslug_enqueue_styles_scripts()
function is hooked into thewp_enqueue_scripts()
action. This ensures that stylesheet and script files are loaded at the right time.
If you’re using a child theme, make sure you replace all instances of get_template_directory_uri()
with get_stylesheet_directory_uri()
.
Also, don’t forget to replace all instances of themeslug
with your actual theme’s slug.
At this point, your lightbox should be fully functional. However, we’re working with WordPress, and the job is not properly done until the Colorbox plugin’s text strings are translation-ready. Let’s handle this last task.
Internationalizing the JQuery Colorbox Text Strings
It’s recommended that all text strings in a WordPress theme or plugin should be internationalized. Internationalization
… is the process of developing your theme, so it can easily be
translated into other languages. Internationalization is often
abbreviated as i18n (because there are 18 letters between the i and
the n).
WordPress uses the gettext libraries for this task. If you’ve worked with WordPress themes or plugins, you’re likely to have come across some of the gettext functions, like __()
and _e()
.
These and other translation functions usually include the text string to be translated as one of their arguments, together with the theme’s or plugin’s textdomain. This is a unique identifier enabling WordPress to distinguish among all the different translations.
jQuery Colorbox has its own text strings. You can find them by opening any of the files in the i18n
folder. We need to make sure these strings are somehow sent to WordPress so that they can be internationalized together with your theme’s text strings. The problem is: how do I transfer data from JavaScript code to WordPress?
The answer lies with a great WordPress function called wp_localize_script()
.
wp_localize_script()
takes three required parameters:
$handle
. This is the handle or identifier for the script where the text strings are located. The data for this parameter is the first argument in thewp_enqueue_script()
function used to enqueue the Colorbox JavaScript file. In this case it’s ‘colorbox
‘;$object_name
. This is an object created by thewp_localize_script()
function, which will be accessible from the JavaScript code inmain.js
;$l10n
. This parameter represents the actual data to be communicated from the JavaScript code to WordPress as an array of key/value pairs.
Let’s see wp_localize_script()
in action. Locate the themeslug_enqueue_styles_scripts()
function you wrote previously in functions.php
. Just below the line of code to enqueue the Colorbox JavaScript file …
wp_enqueue_script( 'colorbox', get_template_directory_uri() .
'/colorbox/jquery.colorbox-min.js', array( 'jquery' ), '', true );
… add the following code:
//Make the Colorbox text translation-ready
$current = 'current';
$total = 'total';
wp_localize_script( 'colorbox', 'themeslug_script_vars', array(
'current' => sprintf(__( 'image {%1$s} of {%2$s}', 'themeslug'), $current, $total ),
'previous' => __( 'previous', 'themeslug' ),
'next' => __( 'next', 'themeslug' ),
'close' => __( 'close', 'themeslug' ),
'xhrError' => __( 'This content failed to load.', 'themeslug' ),
'imgError' => __( 'This image failed to load.', 'themeslug' )
)
);
Regular strings in the code above are internationalized using __('string', 'textdomain')
function. However, current
and total
are not being used as simple text strings. In fact, Colorbox uses them as placeholders to workout the number of the current photo and of the total number of photos respectively. To preserve this functionality, the code above uses the PHP sprintf()
function with appropriate placeholders.
Next, go back to the Colorbox configuration script in main.js
and add this code inside the cbSettings
JavaScript object, after title
:
current: themeslug_script_vars.current,
previous: themeslug_script_vars.previous,
next: themeslug_script_vars.next,
close: themeslug_script_vars.close,
xhrError: themeslug_script_vars.xhrError,
imgError: themeslug_script_vars.imgError
Now the Colorbox configuration settings code looks like this:
//Settings for lightbox
var cbSettings = {
rel: 'cboxElement',
width: '95%',
height: 'auto',
maxWidth: '660',
maxHeight: 'auto',
title: function() {
return $(this).find('img').attr('alt');
},
current: themeslug_script_vars.current,
previous: themeslug_script_vars.previous,
next: themeslug_script_vars.next,
close: themeslug_script_vars.close,
xhrError: themeslug_script_vars.xhrError,
imgError: themeslug_script_vars.imgError
}
Awesome, you’ve just configured the internationalization settings.
Values in each key/value pair use the themeslug_script_vars
object from the wp_localize_script()
function you created earlier. It’s this object that makes the JavaScript variable accessible to PHP.
Conclusion
That’s all, the task of adding lightbox functionality to the native WordPress gallery using jQuery Colorbox has been accomplished.
To check the result, add a gallery to a post or page and select the Media File option from the Link to Gallery Settings. Now access the gallery from the front-end and click on an image. You should be able to see the image pop up with a sleek lightbox effect.
If you’re not happy with the lightbox’ appearance, you can change the CSS code you find in the Colorbox stylesheet using your theme’s style.css
file.
Would you rather have used a WordPress Colorbox plugin or did you enjoy coding up the functionality yourself?
Let me know what you think!
Frequently Asked Questions (FAQs) about Adding a Stylish Lightbox Effect to the WordPress Gallery
What is a Lightbox effect in WordPress?
A Lightbox effect in WordPress is a feature that allows you to overlay images and videos on the current page, thus creating a “lightbox” around them. This effect is commonly used in photo galleries where clicking on a thumbnail image opens up a larger version of the image in a lightbox. It enhances the user experience by allowing users to view images or videos without leaving the current page.
How can I add a Lightbox effect to my WordPress gallery without using a plugin?
While plugins make it easier to add a Lightbox effect to your WordPress gallery, it’s possible to do it manually by modifying your theme’s HTML and CSS. You’ll need to add a specific class to your image link and then use CSS to style the Lightbox effect. However, this requires a good understanding of HTML and CSS. If you’re not comfortable with coding, it’s recommended to use a plugin.
Can I customize the Lightbox effect in WordPress?
Yes, you can customize the Lightbox effect in WordPress. This can be done either through the settings of your Lightbox plugin or by modifying the CSS if you’re adding the Lightbox effect manually. You can change aspects like the animation speed, overlay color, border width, and more.
Why isn’t my Lightbox effect working in WordPress?
There could be several reasons why your Lightbox effect isn’t working. It could be due to a conflict with another plugin, an issue with your theme, or incorrect implementation of the Lightbox effect. Try deactivating other plugins to see if that resolves the issue. If not, check your implementation of the Lightbox effect to ensure it’s correct.
How can I add a Lightbox effect to a single image in WordPress?
To add a Lightbox effect to a single image in WordPress, you’ll need to add a specific class to the image link. This can be done in the HTML editor of your post or page. Once the class is added, the Lightbox effect should work when the image is clicked.
Can I use the Lightbox effect with videos in WordPress?
Yes, you can use the Lightbox effect with videos in WordPress. This can be done by adding a specific class to your video link, similar to how you would with images. When the video link is clicked, the video will open in a Lightbox.
Is it possible to add captions to images in the Lightbox effect?
Yes, it’s possible to add captions to images in the Lightbox effect. This can be done in the media settings of your WordPress dashboard. When you upload an image, you’ll have the option to add a caption. This caption will then appear in the Lightbox when the image is clicked.
Can I add a Lightbox effect to a WordPress gallery created with a page builder?
Yes, you can add a Lightbox effect to a WordPress gallery created with a page builder. The process may vary depending on the page builder you’re using, but generally, you’ll need to add a specific class to your gallery or image links.
How can I disable the Lightbox effect in WordPress?
To disable the Lightbox effect in WordPress, you’ll need to remove the specific class from your image or video links. If you’re using a plugin, you can simply deactivate or uninstall the plugin to disable the Lightbox effect.
Is the Lightbox effect responsive in WordPress?
Yes, the Lightbox effect is responsive in WordPress. This means it will automatically adjust to fit different screen sizes, ensuring a good user experience on both desktop and mobile devices. However, the responsiveness may depend on the specific Lightbox plugin or code you’re using.
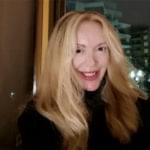
Maria Antonietta Perna is a teacher and technical writer. She enjoys tinkering with cool CSS standards and is curious about teaching approaches to front-end code. When not coding or writing for the web, she enjoys reading philosophy books, taking long walks, and appreciating good food.